C#: Possible Palindromes from string of characters
From Wikipedia
A palindrome is a word, number, phrase, or other sequence of characters which reads the same backward as forward, such as madam or racecar.
Write a C# Sharp program to check whether a given string of characters can be transformed into a palindrome. Return true otherwise false.
Sample Data:
("amamd") -> True
("pamamd") -> False
("ferre") -> True
Sample Solution-1:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
using System.Linq;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Initialize a string variable with some text content
string text = "amamd";
// Display the original string
Console.WriteLine("Original string: " + text);
// Check if the characters in the string can be transformed into a palindrome
Console.WriteLine("Check the said string of characters can be transformed into a palindrome? " + test(text));
// Update the string variable with a different text content
text = "pamamd";
// Display the updated string
Console.WriteLine("\nOriginal string: " + text);
// Check if the characters in the updated string can be transformed into a palindrome
Console.WriteLine("Check the said string of characters can be transformed into a palindrome? " + test(text));
// Update the string variable with another text content
text = "ferre";
// Display the updated string
Console.WriteLine("\nOriginal string: " + text);
// Check if the characters in the updated string can be transformed into a palindrome
Console.WriteLine("Check the said string of characters can be transformed into a palindrome? " + test(text));
}
// Method to check if a string of characters can be transformed into a palindrome
public static bool test(string text)
{
// Concatenate the characters in the string after ordering them
string orderedText = string.Concat(text.OrderBy(x => x));
// Replace the consecutive same characters with an empty string using Regex
// If the length of modified string is less than or equal to 1, it can be a palindrome
bool result = Regex.Replace(orderedText, @"([a-z])\1{1}", string.Empty).Length <= 1;
// Return the result indicating whether the string can be transformed into a palindrome
return result;
}
}
}
Sample Output:
Original string: amamd Check the said string of characters can be transformed into a palindrome? True Original string: pamamd Check the said string of characters can be transformed into a palindrome? False Original string: ferre Check the said string of characters can be transformed into a palindrome? True
Flowchart:
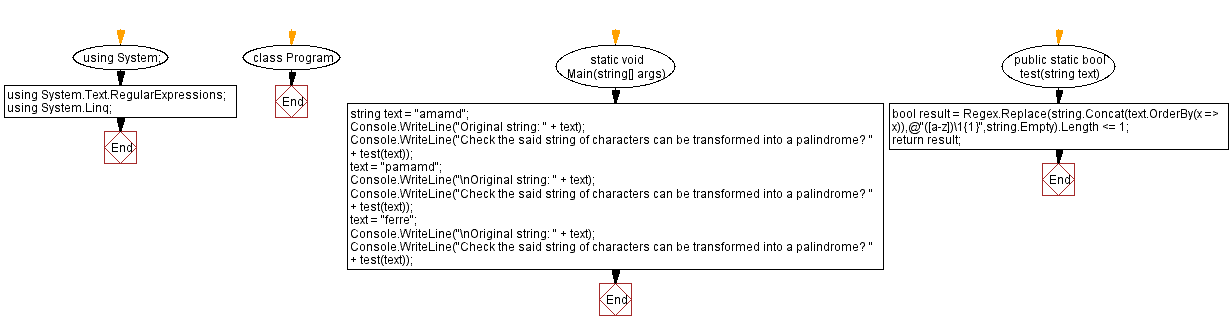
Sample Solution-2:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
using System.Linq;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Initialize a string variable with some text content
string text = "amamd";
// Display the original string
Console.WriteLine("Original string: " + text);
// Check if the characters in the string can be transformed into a palindrome
Console.WriteLine("Check the said string of characters can be transformed into a palindrome? " + test(text));
// Update the string variable with a different text content
text = "pamamd";
// Display the updated string
Console.WriteLine("\nOriginal string: " + text);
// Check if the characters in the updated string can be transformed into a palindrome
Console.WriteLine("Check the said string of characters can be transformed into a palindrome? " + test(text));
// Update the string variable with another text content
text = "ferre";
// Display the updated string
Console.WriteLine("\nOriginal string: " + text);
// Check if the characters in the updated string can be transformed into a palindrome
Console.WriteLine("Check the said string of characters can be transformed into a palindrome? " + test(text));
}
// Method to check if a string of characters can be transformed into a palindrome
public static bool test(string text)
{
// Concatenate the characters in the string after ordering them
text = string.Concat(text.OrderBy(c => c));
// Use Regex to replace consecutive identical characters with an empty string
// If the length of the modified string is less than or equal to 1, it can be a palindrome
bool result = Regex.Replace(text, @"(.)\1", "").Length <= 1;
// Return the result indicating whether the string can be transformed into a palindrome
return result;
}
}
}
Sample Output:
Original string: amamd Check the said string of characters can be transformed into a palindrome? True Original string: pamamd Check the said string of characters can be transformed into a palindrome? False Original string: ferre Check the said string of characters can be transformed into a palindrome? True
Flowchart:
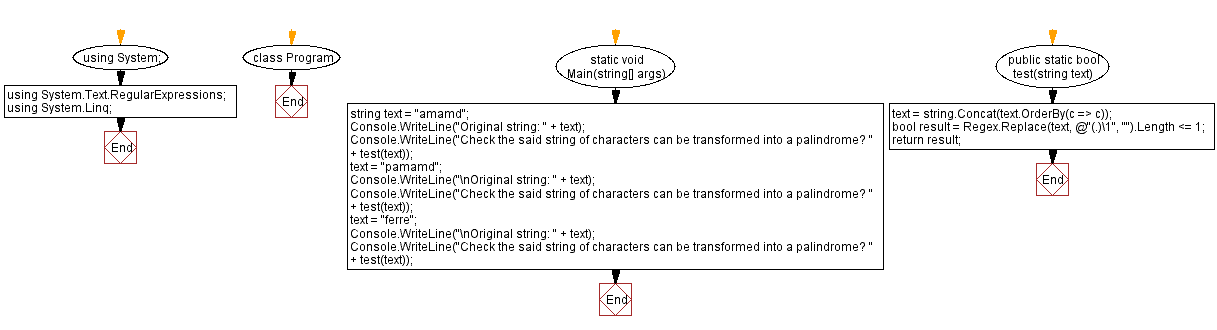
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Calculate the average word length in a string.
Next C# Sharp Exercise: Validate a password.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics