C#: Calculate the average word length in a string
Write a C# Sharp program to calculate the average word length in a given string. Round the average length up to two decimal places.
Note: Ignore punctuation.
Sample Data:
("CPP Exercises." -> 6
("C# syntax is highly expressive, yet it is also simple and easy to learn.") -> 4
(“C# is an elegant and type-safe object-oriented language") -> 5.62
Sample Solution-1:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
using System.Linq;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Initialize a string variable with some text content
string text = "CPP Exercises.";
// Display the original string
Console.WriteLine("Original string: " + text);
// Calculate and display the average word length in the string
Console.WriteLine("The average word length in the said string: " + test(text));
// Update the string variable with a different text content
text = "C# syntax is highly expressive, yet it is also simple and easy to learn.";
// Display the updated string
Console.WriteLine("Original string: " + text);
// Calculate and display the average word length in the updated string
Console.WriteLine("The average word length in the said string: " + test(text));
// Update the string variable with another text content
text = "C# is an elegant and type-safe object-oriented language";
// Display the updated string
Console.WriteLine("Original string: " + text);
// Calculate and display the average word length in the updated string
Console.WriteLine("The average word length in the said string: " + test(text));
}
// Method to calculate the average word length in a string
public static double test(string text)
{
// Remove non-alphabetic characters and keep spaces to isolate words
string new_text = Regex.Replace(text, "[^A-Za-z ]", "");
// Split the string into words, calculate the length of each word, find the average length of all words
double average_len = new_text.Split(' ').Select(x => x.Length).Average();
// Round the average word length to 2 decimal places and return the result
return Math.Round(average_len, 2);
}
}
}
Sample Output:
Original string: CPP Exercises. The average word length in the said string: 6 Original string: C# syntax is highly expressive, yet it is also simple and easy to learn. The average word length in the said string: 4 Original string: C# is an elegant and type-safe object-oriented language The average word length in the said string: 5.62
Flowchart:
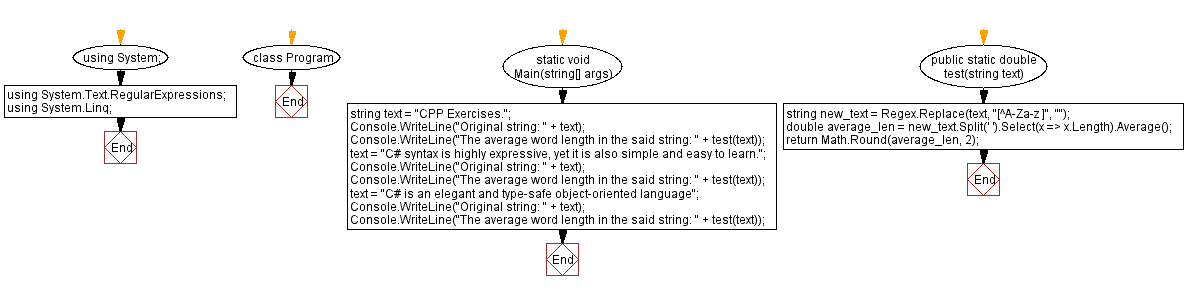
Sample Solution-2:
C# Sharp Code:
using System;
using System.Text.RegularExpressions;
using System.Linq;
namespace exercises
{
class Program
{
// Main method - entry point of the program
static void Main(string[] args)
{
// Initialize a string variable with some text content
string text = "CPP Exercises.";
// Display the original string
Console.WriteLine("Original string: " + text);
// Calculate and display the average word length in the string
Console.WriteLine("The average word length in the said string: " + test(text));
// Update the string variable with a different text content
text = "C# syntax is highly expressive, yet it is also simple and easy to learn.";
// Display the updated string
Console.WriteLine("Original string: " + text);
// Calculate and display the average word length in the updated string
Console.WriteLine("The average word length in the said string: " + test(text));
// Update the string variable with another text content
text = "C# is an elegant and type-safe object-oriented language";
// Display the updated string
Console.WriteLine("Original string: " + text);
// Calculate and display the average word length in the updated string
Console.WriteLine("The average word length in the said string: " + test(text));
}
// Method to calculate the average word length in a string
public static double test(string text)
{
// Count the number of letters in the string
int letterCount = text.Where(ch => char.IsLetter(ch)).Count();
// Count the number of white spaces in the string and add 1 (for the last word)
int wordCount = text.Where(ch => char.IsWhiteSpace(ch)).Count() + 1;
// Calculate and return the average word length by dividing the total letters by the total words
return Math.Round((double)letterCount / (double)wordCount, 2);
}
}
}
Sample Output:
Original string: CPP Exercises. The average word length in the said string: 6 Original string: C# syntax is highly expressive, yet it is also simple and easy to learn. The average word length in the said string: 4 Original string: C# is an elegant and type-safe object-oriented language The average word length in the said string: 5.62
Flowchart:
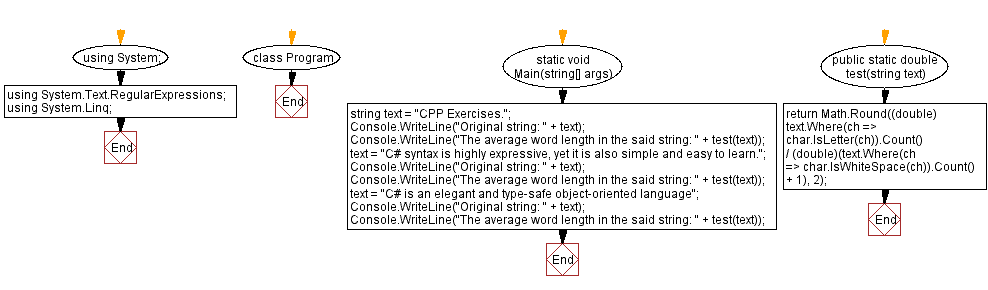
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous C# Sharp Exercise: Check a string is Valid Hex Code or not.
Next C# Sharp Exercise: Possible Palindromes from string of characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics