C#: Least Common Multiple (LCM) of more than two numbers
Write a C# Sharp program to find the Least Common Multiple (LCM) of more than two numbers. Take numbers from a given array of positive integers.
From Wikipedia,
In arithmetic and number theory, the least common multiple, lowest common multiple, or smallest common multiple of two integers a and b, usually denoted by lcm(a, b), is the smallest positive integer that is divisible by both a and b. Since division of integers by zero is undefined, this definition has meaning only if a and b are both different from zero. However, some authors define lcm(a,0) as 0 for all a, which is the result of taking the lcm to be the least upper bound in the lattice of divisibility.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
static void Main(string[] args)
{
int[] nums1 = { 4, 6, 8 };
Console.WriteLine("Original array elements:");
for (int i = 0; i < nums1.Length; i++)
{
Console.Write(nums1[i] + " ");
}
Console.WriteLine("\nLCM of the numbers of the said array of positive integers: " + test(nums1));
int[] nums2 = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
Console.WriteLine("\nOriginal array elements:");
for (int i = 0; i < nums2.Length; i++)
{
Console.Write(nums2[i] + " ");
}
Console.WriteLine("\nLCM of the numbers of the said array of positive integers: " + test(nums2));
int[] nums3 = { 48, 72, 108 };
Console.WriteLine("\nOriginal array elements:");
for (int i = 0; i < nums3.Length; i++)
{
Console.Write(nums3[i] + " ");
}
Console.WriteLine("\nLCM of the numbers of the said array of positive integers: " + test(nums3));
}
static int gcd(int n1, int n2)
{
if (n2 == 0)
{
return n1;
}
else
{
return gcd(n2, n1 % n2);
}
}
public static int test(int[] numbers)
{
return numbers.Aggregate((S, val) => S * val / gcd(S, val));
}
}
}
Sample Output:
Original array elements: 4 6 8 LCM of the numbers of the said array of positive integers: 24 Original array elements: 1 2 3 4 5 6 7 8 9 10 LCM of the numbers of the said array of positive integers: 2520 Original array elements: 48 72 108 LCM of the numbers of the said array of positive integers: 432
Flowchart:
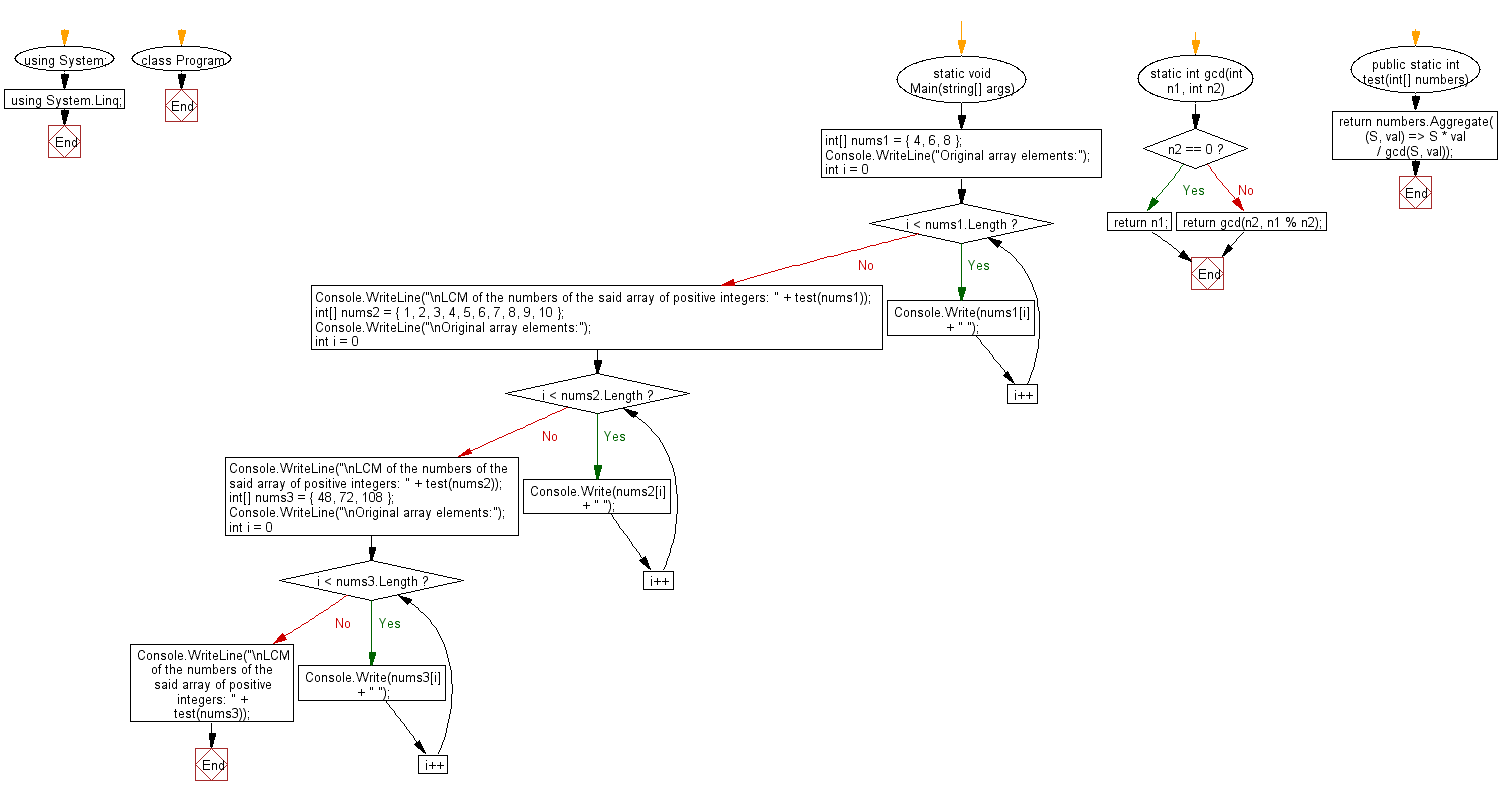
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to find the value of PI up to n (given number) decimals places. Next: Write a C# Sharp program to get the nth tetrahedral number from a given integer(n) value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics