C#: Reverse the digits of a given signed 32-bit integer
Write a C# Sharp program to reverse the digits of a 32-bit signed integer.
Sample Solution:
C# Sharp Code:
Sample Output:
Original Integer value: 123456 Reverse the digits of the said signed integer value: 654321 Original Integer value: -7654 Reverse the digits of the said signed integer value: -4567 Original Integer value: 100 Reverse the digits of the said signed integer value: 1
Flowchart:
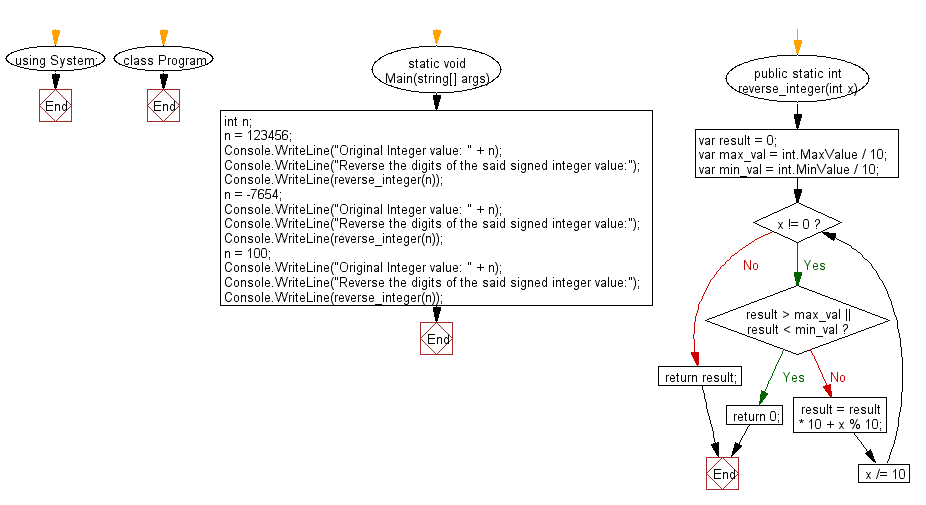
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to calculate the full product of two 32-bit numbers.
Next: Write a C# Sharp program to convert a given string value to a 32-bit signed integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.