C#: Create a list of numbers and display the numbers greater than 80
Write a program in C# Sharp to create a list of numbers and display numbers greater than 80.
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
class LinqExercise9
{
static void Main(string[] args)
{
int i = 0; // Initializing an integer variable i with value 0
List<int> templist = new List<int>(); // Creating a new list of integers named templist
// Adding integers to the templist
templist.Add(55);
templist.Add(200);
templist.Add(740);
templist.Add(76);
templist.Add(230);
templist.Add(482);
templist.Add(95);
Console.Write("\nLINQ : Create a list of numbers and display the numbers greater than 80 : ");
Console.Write("\n-------------------------------------------------------------------------\n");
Console.WriteLine("\nThe members of the list are : ");
// Displaying the members of the templist using foreach loop
foreach (var lstnum in templist)
{
Console.Write(lstnum + " ");
}
// Creating a new list named FilterList with numbers greater than 80 using FindAll method
List<int> FilterList = templist.FindAll(x => x > 80 ? true : false);
Console.WriteLine("\n\nThe numbers greater than 80 are : ");
// Displaying numbers in FilterList greater than 80 using foreach loop
foreach (var num in FilterList)
{
Console.WriteLine(num);
}
Console.ReadLine(); // Allowing the user to view the output before closing the console
}
}
Sample Output:
LINQ : Create a list of numbers and display the numbers greater than 80 : ------------------------------------------------------------------------- The members of the list are : 55 200 740 76 230 482 95 The numbers greater than 80 are : 200 740 230 482 95
Visual Presentation:
Flowchart:
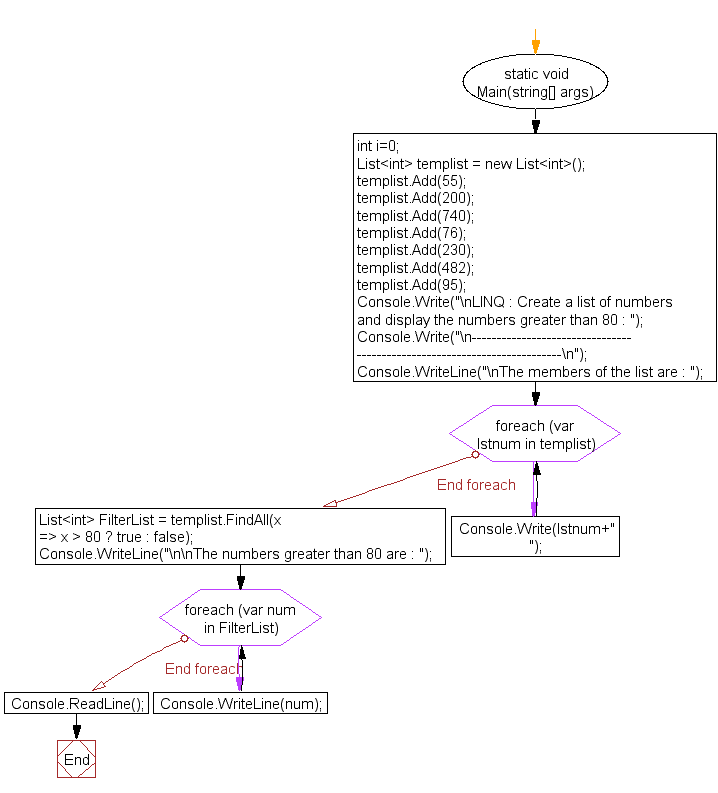
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the string which starts and ends with a specific character.
Next: Write a program in C# Sharp to Accept the members of a list through the keyboard and display the members more than a specific value.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.