C#: Find the string which starts and ends with a specific character
Write a program in C# Sharp to find a string that starts and ends with a specific character.
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
class LinqExercise8
{
static void Main(string[] args)
{
string chst, chen; // Declaring variables to store starting and ending characters
char ch; // Declaring a variable to temporarily store a character input
string[] cities = // Array containing city names
{
"ROME", "LONDON", "NAIROBI", "CALIFORNIA", "ZURICH", "NEW DELHI", "AMSTERDAM", "ABU DHABI", "PARIS"
};
Console.Write("\nLINQ : Find the string which starts and ends with a specific character : ");
Console.Write("\n-----------------------------------------------------------------------\n");
Console.Write("\nThe cities are : 'ROME','LONDON','NAIROBI','CALIFORNIA','ZURICH','NEW DELHI','AMSTERDAM','ABU DHABI','PARIS' \n");
Console.Write("\nInput starting character for the string : ");
ch = (char)Console.Read(); // Accepting input for starting character
chst = ch.ToString(); // Converting the character input to a string
Console.Write("\nInput ending character for the string : ");
ch = (char)Console.Read(); // Accepting input for ending character
chen = ch.ToString(); // Converting the character input to a string
var _result = from x in cities // LINQ query to filter cities based on starting and ending characters
where x.StartsWith(chst)
where x.EndsWith(chen)
select x;
Console.Write("\n\n");
foreach(var city in _result) // Looping through the filtered cities and displaying them
{
Console.Write("The city starting with {0} and ending with {1} is : {2} \n", chst, chen, city);
}
Console.ReadLine(); // Allowing the user to view the output before closing the console
}
}
Sample Output:
LINQ : Find the string which starts and ends with a specific character : ----------------------------------------------------------------------- The cities are : 'ROME','LONDON','NAIROBI','CALIFORNIA','ZURICH','NEW DELHI','AMSTERDAM','ABU DHABI','PARIS' Input starting character for the string : N Input ending character for the string : I The city starting with N and ending with I is : NAIROBI The city starting with N and ending with I is : NEW DELHI
Visual Presentation:
Flowchart:
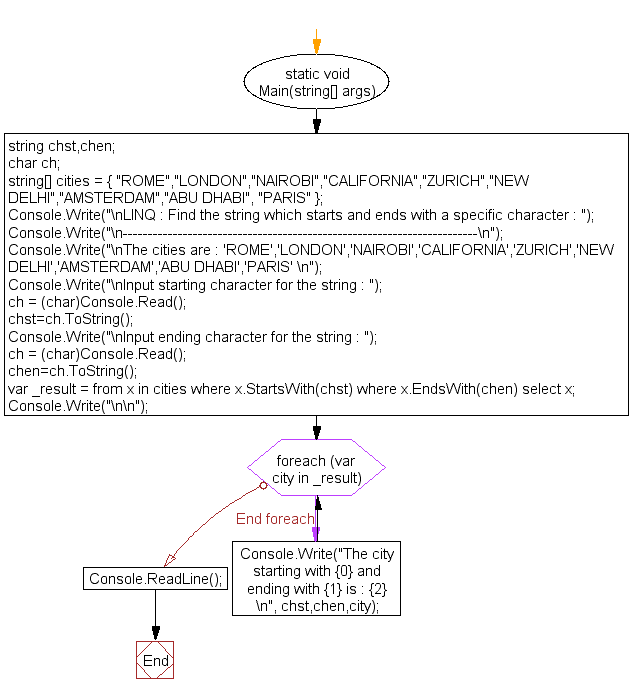
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display numbers, multiplication of number with frequency and the frequency of number of giving array.
Next: Write a program in C# Sharp to create a list of numbers and display the numbers greater than 80 as output.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.