C#: Display numbers, number*frequency and the frequency
Write a program in C# Sharp to display numbers, multiplication of numbers with frequency and the frequency of a number in an array.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise7
{
static void Main(string[] args)
{
// Define an array containing integer numbers
int[] nums = new int[] { 5, 1, 9, 2, 3, 7, 4, 5, 6, 8, 7, 6, 3, 4, 5, 2 };
// Display a message indicating the purpose of the LINQ operation
Console.Write("\nLINQ : Display numbers, number*frequency and frequency : ");
Console.Write("\n-------------------------------------------------------\n");
Console.Write("The numbers in the array are : \n");
Console.Write(" 5, 1, 9, 2, 3, 7, 4, 5, 6, 8, 7, 6, 3, 4, 5, 2 \n\n");
// Group the array elements by their values and count occurrences
var m = from x in nums
group x by x into y
select y;
// Display the headers for the output table
Console.Write("Number"+"\t"+"Number*Frequency"+"\t"+"Frequency"+"\n");
Console.Write("------------------------------------------------\n");
// Display the number, its product with frequency, and frequency
foreach (var arrEle in m)
{
Console.WriteLine(arrEle.Key + "\t" + arrEle.Sum() + "\t\t\t" + arrEle.Count());
}
Console.WriteLine();
}
}
Sample Output:
LINQ : Display numbers, number*frequency and frequency : ------------------------------------------------------- The numbers in the array are : 5, 1, 9, 2, 3, 7, 4, 5, 6, 8, 7, 6, 3, 4, 5, 2 Number Number*Frequency Frequency ------------------------------------------------ 5 15 3 1 1 1 9 9 1 2 4 2 3 6 2 7 14 2 4 8 2 6 12 2 8 8 1
Visual Presentation:
Flowchart:
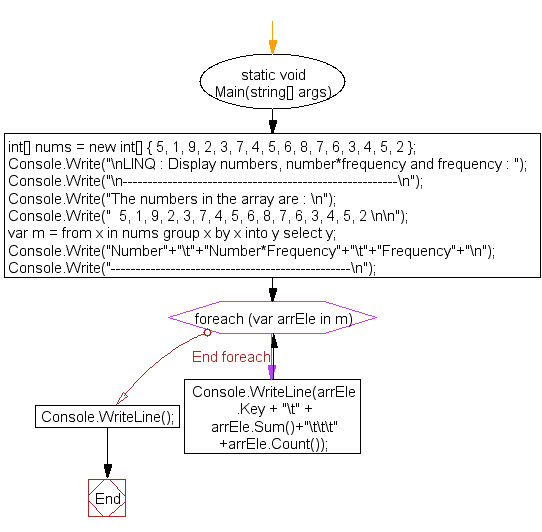
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the name of the days of a week.
Next: Write a program in C# Sharp to find the string which starts and ends with a specific character.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.