C#: Display the characters and frequency of character from giving string
Write a program in C# Sharp to display the characters and frequency of each character in a given string.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise5
{
static void Main(string[] args)
{
string str; // Declare a string variable to store user input
// Display a message prompting the user to input a string
Console.Write("\nLINQ : Display the characters and frequency of character from giving string : ");
Console.Write("\n----------------------------------------------------------------------------\n");
Console.Write("Input the string : ");
// Read the string input from the user
str = Console.ReadLine();
Console.Write("\n");
// Group the characters in the string by their values and count occurrences
var FreQ = from x in str
group x by x into y
select y;
// Display the frequency of characters
Console.Write("The frequency of the characters are :\n");
foreach(var ArrEle in FreQ)
{
Console.WriteLine("Character " + ArrEle.Key + ": " + ArrEle.Count() + " times");
}
}
}
Sample Output:
LINQ : Display the characters and frequency of character from giving string : ---------------------------------------------------------------------------- Input the string : w3resource The frequency of the characters are : Character w: 1 times Character 3: 1 times Character r: 2 times Character e: 2 times Character s: 1 times Character o: 1 times Character u: 1 times Character c: 1 times
Visual Presentation:
Flowchart:
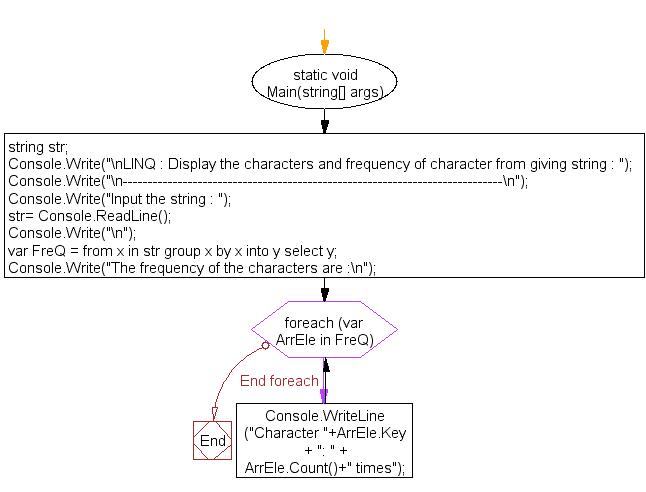
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the number and frequency of number from given array.
Next: Write a program in C# Sharp to display the name of the days of a week.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics