C#: Find the number and its square of an array which is more than 20
Write a program in C# Sharp to find the number of an array and the square of each number which is more than 20.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise3
{
static void Main(string[] args)
{
// Define an integer array
var arr1 = new[] { 3, 9, 2, 8, 6, 5 };
// Display a message indicating the purpose of the LINQ operation
Console.Write("\nLINQ : Find the number and its square of an array which is more than 20 : ");
Console.Write("\n------------------------------------------------------------------------\n");
// Create a LINQ query to find numbers and their squares greater than 20
var sqNo = from int Number in arr1
let SqrNo = Number * Number
where SqrNo > 20
select new { Number, SqrNo };
// Iterate through the result and display numbers and their squares
foreach (var a in sqNo)
Console.WriteLine(a);
Console.ReadLine(); // Wait for user input before closing the program
}
}
Sample Output:
LINQ : Find the number and its square of an array which is more than 20 : ------------------------------------------------------------------------ { Number = 9, SqrNo = 81 } { Number = 8, SqrNo = 64 } { Number = 6, SqrNo = 36 } { Number = 5, SqrNo = 25 }
Visual Presentation:
Flowchart:
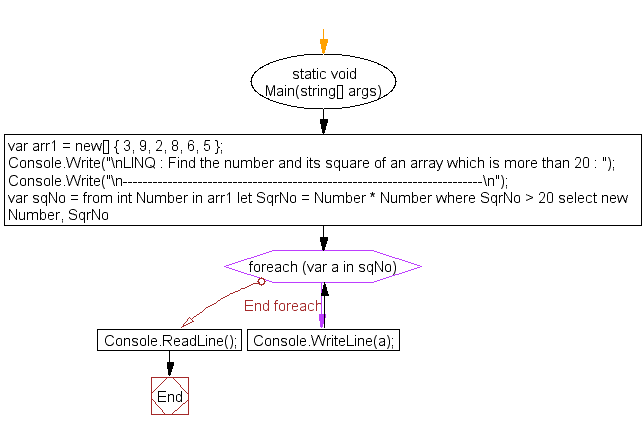
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the positive numbers from a list of numbers using two where conditions in LINQ Query.
Next: Write a program in C# Sharp to display the number and frequency of number from given array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.