C#: Split a collection of strings into some groups
Write a program in C# Sharp to split a collection of strings into some groups.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
class LinqExercise29
{
static void Main(string[] args)
{
// Array of cities
string[] cities =
{
"ROME","LONDON","NAIROBI","CALIFORNIA",
"ZURICH","NEW DELHI","AMSTERDAM",
"ABU DHABI", "PARIS","NEW YORK"
};
// Displaying the instruction for the operation
Console.Write("\nLINQ : Split a collection of strings into some groups : ");
Console.Write("\n-------------------------------------------------------\n");
Console.Write("\nThe cities are : 'ROME','LONDON','NAIROBI','CALIFORNIA','ZURICH','NEW DELHI', \n");
Console.Write(" 'AMSTERDAM','ABU DHABI','PARIS','NEW YORK' \n");
Console.Write("\nHere is the group of cities : \n\n");
// Using LINQ to split the array of cities into groups of three
var citySplit = from i in Enumerable.Range(0, cities.Length)
group cities[i] by i / 3;
// Iterating through each group of cities and displaying them using the cityView method
foreach (var city in citySplit)
{
// Calling cityView method to display each group of cities
cityView(string.Join("; ", city.ToArray()));
}
Console.ReadLine(); // Wait for user input before closing the console
}
// Method to display a group of cities
static void cityView(string cityMetro)
{
Console.WriteLine(cityMetro); // Displaying the group of cities
Console.WriteLine("-- here is a group of cities --\n"); // Additional separator
}
}
Sample Output:
LINQ : Split a collection of strings into some groups : ------------------------------------------------------- The cities are : 'ROME','LONDON','NAIROBI','CALIFORNIA','ZURICH','NEW DELHI', 'AMSTERDAM','ABU DHABI','PARIS','NEW YORK' Here is the group of cities : ROME; LONDON; NAIROBI -- here is a group of cities -- CALIFORNIA; ZURICH; NEW DELHI -- here is a group of cities -- AMSTERDAM; ABU DHABI; PARIS -- here is a group of cities -- NEW YORK -- here is a group of cities --
Visual Presentation:
Flowchart:
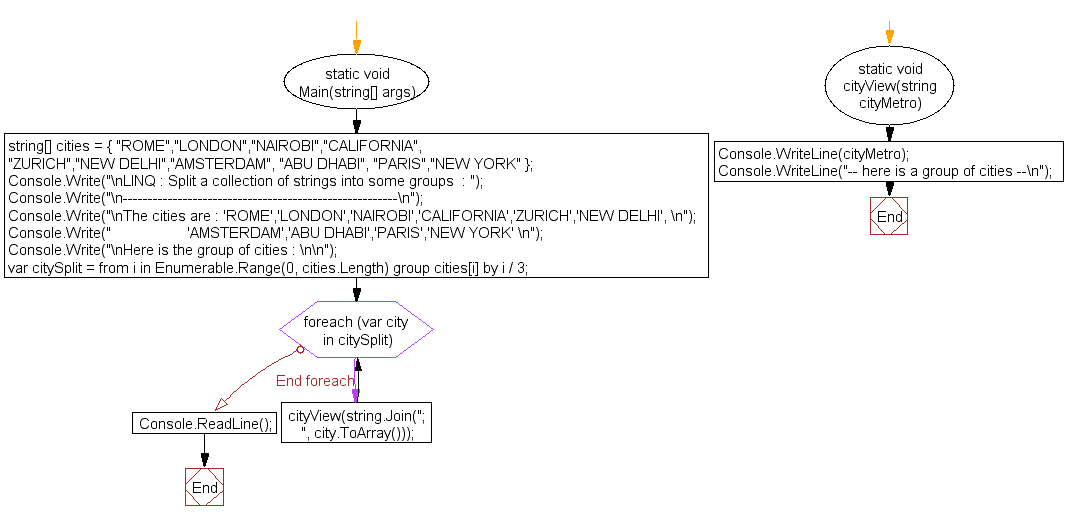
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the list of items in the array according to the length of the string then by name in ascending order.
Next: Write a program in C# Sharp to arrange the distinct elements in the list in ascending order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.