C#: Count file extensions and group it
Write a program in C# Sharp to count file extensions and group it using LINQ.
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.IO;
// Define a class named LinqExercise15
class LinqExercise15
{
// Main method, the entry point of the program
public static void Main()
{
// Initialize an array of file names with different extensions
string[] arr1 = { "aaa.frx", "bbb.TXT", "xyz.dbf", "abc.pdf", "aaaa.PDF", "xyz.frt", "abc.xml", "ccc.txt", "zzz.txt" };
// Display information about the program
Console.Write("\nLINQ : Count file extensions and group it : ");
Console.Write("\n------------------------------------------\n");
// Display the files present in the array
Console.Write("\nThe files are : aaa.frx, bbb.TXT, xyz.dbf,abc.pdf");
Console.Write("\n aaaa.PDF,xyz.frt, abc.xml, ccc.txt, zzz.txt\n");
Console.Write("\nHere is the group of extension of the files : \n\n");
// Using LINQ, group file extensions and count their occurrences
var fGrp = arr1.Select(file => Path.GetExtension(file).TrimStart('.').ToLower())
.GroupBy(z => z, (fExt, extCtr) => new
{
Extension = fExt,
Count = extCtr.Count()
});
// Iterate through the grouped file extensions and display the count of each extension
foreach (var m in fGrp)
Console.WriteLine("{0} File(s) with {1} Extension ", m.Count, m.Extension);
Console.ReadLine(); // Wait for user input before closing the program
}
}
Sample Output:
LINQ : Count File Extensions and Group it : ------------------------------------------ The files are : aaa.frx, bbb.TXT, xyz.dbf,abc.pdf aaaa.PDF,xyz.frt, abc.xml, ccc.txt, zzz.txt Here is the group of extension of the files : 1 File(s) with .frx Extension 3 File(s) with .txt Extension 1 File(s) with .dbf Extension 2 File(s) with .pdf Extension 1 File(s) with .frt Extension 1 File(s) with .xml Extension
Visual Presentation:
Flowchart:
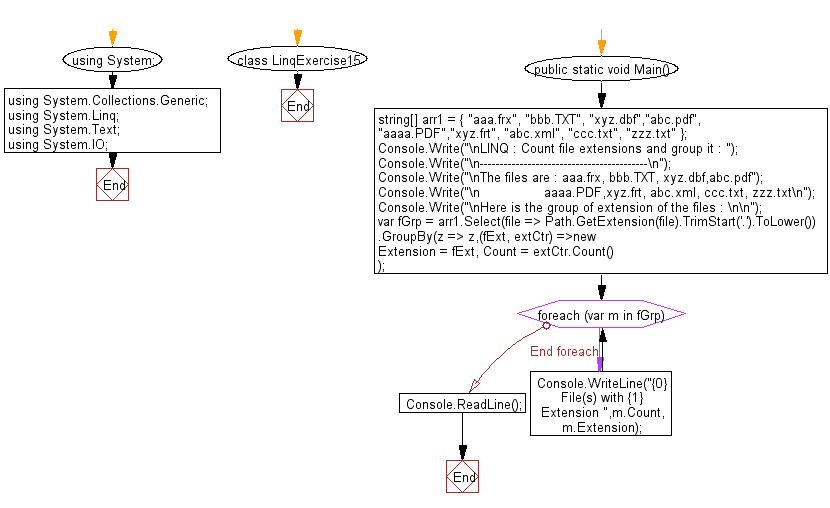
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the n-th Maximum grade point achieved by the students from the list of students.
Next: Write a program in C# Sharp to Calculate Size of File using LINQ.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.