C#: Find the uppercase words in a string
Write a program in C# Sharp to find uppercase words in a string.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.Collections.Generic;
// Define a class named LinqExercise12
class LinqExercise12
{
// Main method, the entry point of the program
static void Main(string[] args)
{
// Display information about the program
Console.Write("\nLINQ : Find the uppercase words in a string : ");
Console.Write("\n----------------------------------------------\n");
string strNew; // Declare a string variable named strNew
// Ask the user to input a string
Console.Write("Input the string : ");
strNew = Console.ReadLine();
// Call the WordFilt method to filter uppercase words in the input string
var ucWord = WordFilt(strNew);
// Display the uppercase words found in the input string
Console.Write("\nThe UPPER CASE words are :\n ");
foreach (string strRet in ucWord)
{
Console.WriteLine(strRet);
}
Console.ReadLine(); // Wait for user input before closing the program
}
// Define a method named WordFilt that takes a string parameter and returns IEnumerable<string>
static IEnumerable<string> WordFilt(string mystr)
{
// Split the input string into words, filter and retrieve uppercase words
var upWord = mystr.Split(' ')
.Where(x => String.Equals(x, x.ToUpper(),
StringComparison.Ordinal));
return upWord; // Return the uppercase words
}
}
Sample Output:
LINQ : Find the uppercase words in a string : ---------------------------------------------- Input the string : this IS a STRING The UPPER CASE words are : IS STRING
Visual Presentation:
Flowchart:
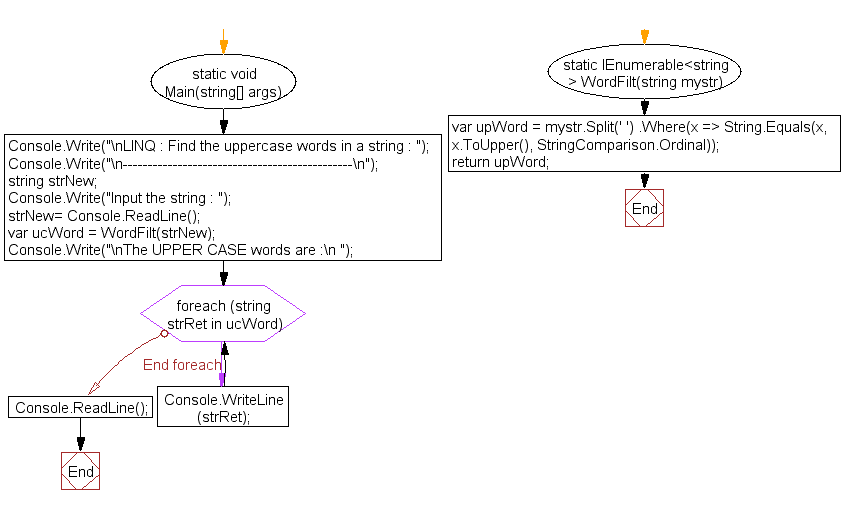
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the top nth records.
Next: Write a program in C# Sharp to convert a string array to a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.