C#: Accept the members of a list and display the members more than a specific value
Write a program in C# Sharp to accept list members through the keyboard and display them more than a specific value.
Sample Solution:
C# Sharp Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
class LinqExercise10
{
static void Main(string[] args)
{
int i = 0; // Initializing a variable i with value 0
int memlist, n, m; // Declaring variables to store list members, count, and comparison value
List<int> templist = new List<int>(); // Creating a new list of integers named templist
Console.Write("\nLINQ : Accept the members of a list and display the members more than a specific value : ");
Console.Write("\n----------------------------------------------------------------------------------------\n");
Console.Write("Input the number of members on the List : ");
n = Convert.ToInt32(Console.ReadLine()); // Accepting the number of members for the list
// Loop to input list members based on user input count 'n'
for (i = 0; i < n; i++)
{
Console.Write("Member {0} : ", i);
memlist = Convert.ToInt32(Console.ReadLine());
templist.Add(memlist); // Adding each member to the templist
}
Console.Write("\nInput the value above which you want to display the members of the List : ");
m = Convert.ToInt32(Console.ReadLine()); // Accepting a value to filter the list
// Creating FilterList to store numbers greater than 'm' using FindAll method
List<int> FilterList = templist.FindAll(x => x > m ? true : false);
Console.WriteLine("\nThe numbers greater than {0} are : ", m);
// Displaying numbers in FilterList greater than 'm' using foreach loop
foreach (var num in FilterList)
{
Console.WriteLine(num);
}
Console.ReadLine(); // Allowing the user to view the output before closing the console
}
}
Sample Output:
LINQ : Accept the members of a list and display the members more than a specific value : ---------------------------------------------------------------------------------------- Input the number of members on the List : 5 Member 0 : 10 Member 1 : 20 Member 2 : 30 Member 3 : 40 Member 4 : 50 Input the value above you want to display the members of the List : 20 The numbers greater than 20 are : 30 40 50
Visual Presentation:
Flowchart:
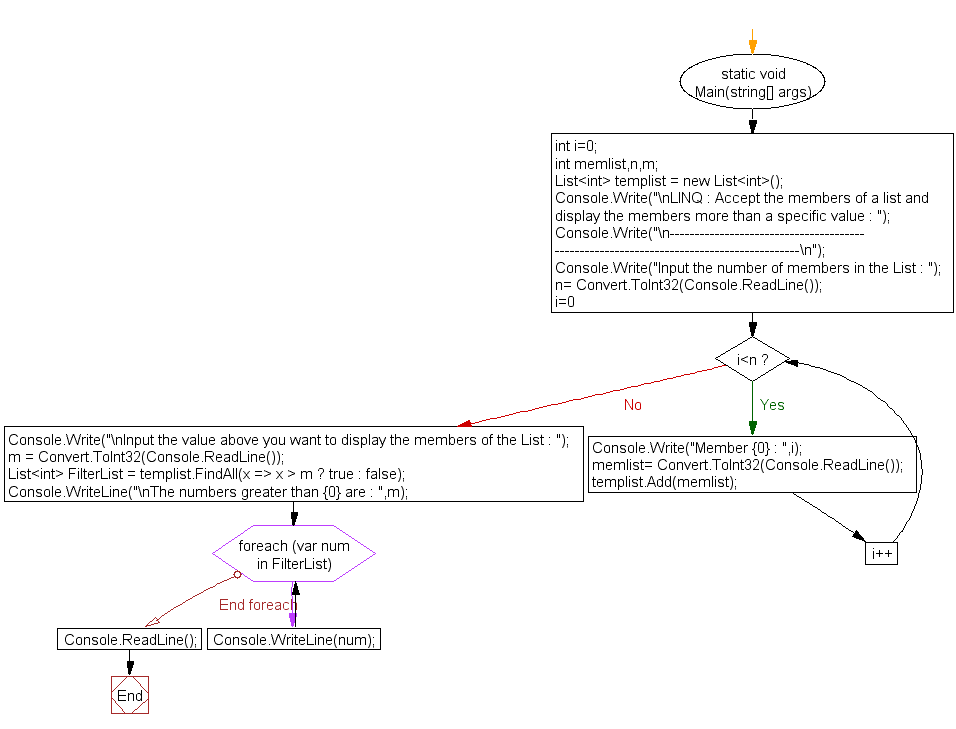
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to create a list of numbers and display the numbers greater than 80 as output.
Next: Write a program in C# Sharp to display the top nth records.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.