C#: To check a number is prime or not
Write a program in C# Sharp to create a function to check whether a number is prime or not.
Visual Presentation:
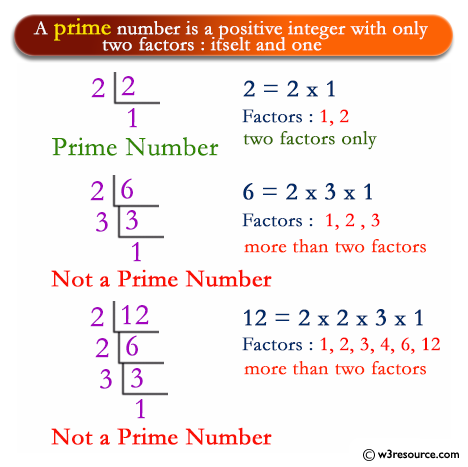
Sample Solution:
C# Sharp Code:
using System;
// Class definition named 'funcexer9'
public class funcexer9
{
// Method to check if a number is prime or not
public static bool chkprime(int num)
{
// Loop to check for factors of the number
for (int i = 2; i < num; i++)
{
if (num % i == 0)
{
return false; // If any factor found, the number is not prime, return false
}
}
return true; // If no factors found, the number is prime, return true
}
// Main method, the entry point of the program
public static void Main()
{
// Display a description of the program
Console.Write("\n\nFunction : To check a number is prime or not :\n");
Console.Write("--------------------------------------------------\n");
// Prompt the user to input a number
Console.Write("Input a number : ");
int n = Convert.ToInt32(Console.ReadLine());
// Check if the number is prime using the 'chkprime' method
if (chkprime(n))
{
Console.WriteLine(n + " is a prime number"); // Display if the number is prime
}
else
{
Console.WriteLine(n + " is not a prime number"); // Display if the number is not prime
}
}
}
Sample Output:
Function : To check a number is prime or not : -------------------------------------------------- Input a number : 23 23 is a prime number
Flowchart :
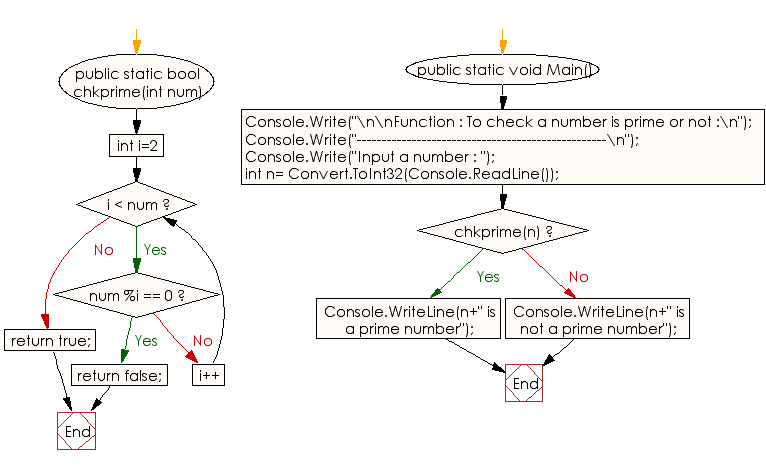
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to create a function to display the n number Fibonacci sequence.
Next: Write a program in C# Sharp to create a function to calculate the sum of the individual digits of a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.