C#: To display the n number Fibonacci sequence
Write a program in C# Sharp to create a function to display the n number Fibonacci sequence.
Visual Presentation:
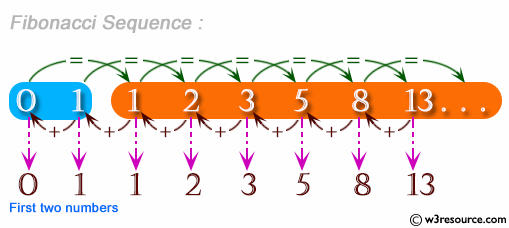
Sample Solution:
C# Sharp Code:
using System;
// Class definition named 'funcexer8'
class funcexer8
{
// Method to calculate the Fibonacci series up to 'nno' numbers
public static int Fibo(int nno)
{
int num1 = 0;
int num2 = 1;
// Loop to calculate Fibonacci series
for (int i = 0; i < nno; i++)
{
int temp = num1;
num1 = num2;
num2 = temp + num2;
}
return num1; // Return the Fibonacci number
}
// Main method, the entry point of the program
public static void Main()
{
// Display a description of the program
Console.Write("\n\nFunction : To display the n number Fibonacci series :\n");
Console.Write("------------------------------------------------------------\n");
// Prompt the user to input the number of Fibonacci Series
Console.Write("Input number of Fibonacci Series : ");
int n = Convert.ToInt32(Console.ReadLine());
Console.WriteLine("The Fibonacci series of " + n + " numbers is :");
// Loop to print the Fibonacci series up to 'n' numbers
for (int i = 0; i < n; i++)
{
Console.Write(Fibo(i) + " "); // Call the 'Fibo' method to calculate and display each Fibonacci number
}
Console.WriteLine(); // Move to the next line after displaying the series
}
}
Sample Output:
Function : To display the n number Fibonacci series : ------------------------------------------------------------ Input number of Fibonacci Series : 10 The Fibonacci series of 10 numbers is : 0 1 1 2 3 5 8 13 21 34
Flowchart:
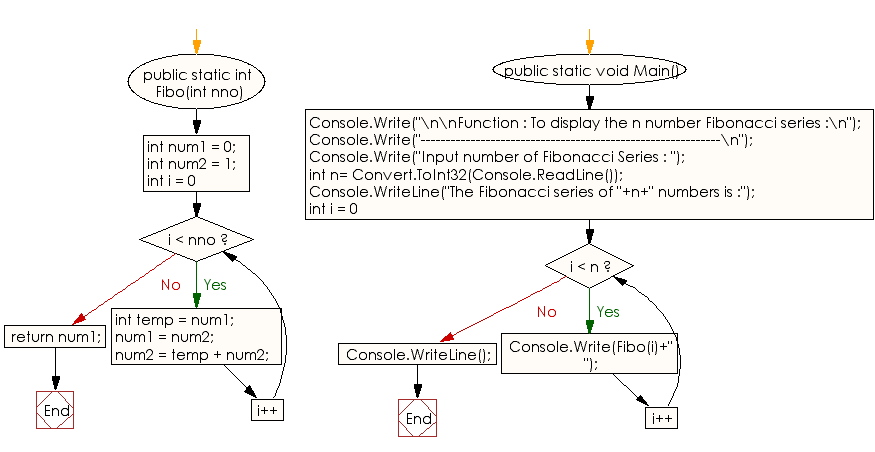
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to create a function to calculate the result of raising an integer number to another.
Next: Write a program in C# Sharp to create a function to check whether a number is prime or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics