C#: To calculate the result of raising an integer number to another
Write a program in C# Sharp to create a function to calculate the result of raising an integer number to another.
Visual Presentation:
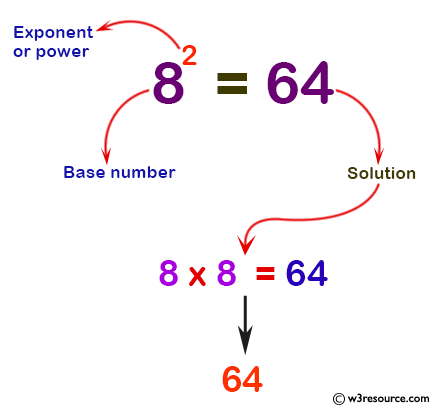
Sample Solution:
C# Sharp Code:
using System;
// Define a public class named 'funcexer7'
public class funcexer7
{
// Main method, the entry point of the program
public static void Main()
{
int n1;
int exp1;
// Display a description of the program
Console.Write("\n\nFunction : To calculate the result of raising an integer number to another :\n");
Console.Write("--------------------------------------------------------------------------------\n");
// Prompt the user to input the base number
Console.Write("Input Base number: ");
n1 = Convert.ToInt32(Console.ReadLine());
// Prompt the user to input the exponent
Console.Write("Input the Exponent : ");
exp1 = Convert.ToInt32(Console.ReadLine());
// Calculate and display the result of raising 'n1' to the power of 'exp1'
Console.WriteLine("So, the number {0} ^ (to the power) {1} = {2} ", n1, exp1, PowerRaising(n1, exp1));
}
// Define a method 'PowerRaising' to calculate the power of a number
public static int PowerRaising(int num, int exp)
{
int rvalue = 1;
int i;
// Calculate the power by iterating 'exp' times
for (i = 1; i <= exp; i++)
{
rvalue = rvalue * num; // Update the result 'rvalue' by multiplying 'num'
}
return rvalue; // Return the calculated result
}
}
Sample Output:
Function : To calculate the result of raising an integer number to another : -------------------------------------------------------------------------------- Input Base number: 8 Input the Exponent : 2 So, the number 8 ^ (to the power) 2 = 64
Flowchart :
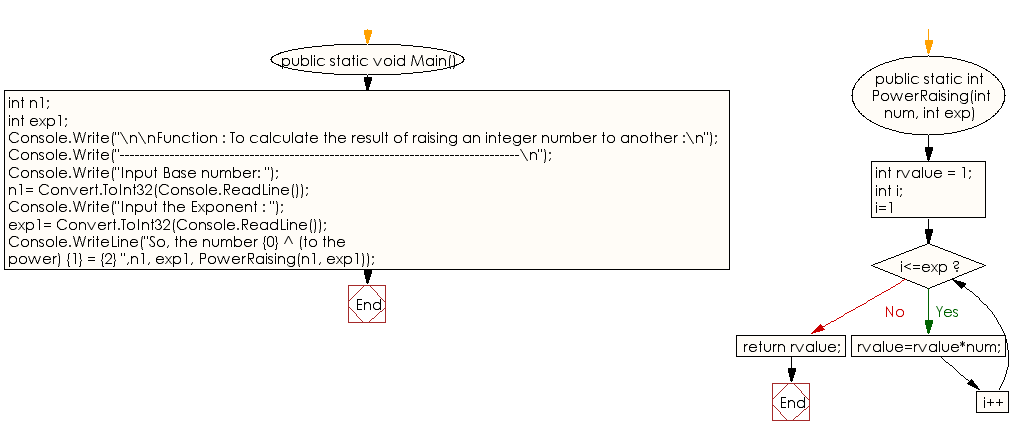
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to create a function to swap the values of two integer numbers.
Next: Write a program in C# Sharp to create a function to display the n number Fibonacci sequence.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.