C#: Function to calculate the sum of two numbers
Write a program in C# Sharp to create a function for the sum of two numbers.
Visual Presentation:
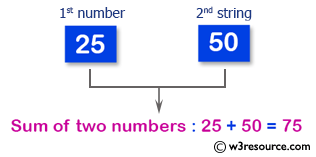
Sample Solution:
C# Sharp Code:
using System;
// Define a public class named 'funcexer3'
public class funcexer3
{
// Define a public static method 'Sum' that takes two integer parameters and returns their sum
public static int Sum(int num1, int num2)
{
int total;
total = num1 + num2;
return total;
}
// Main method, the entry point of the program
public static void Main()
{
// Print a description of the program
Console.Write("\n\nFunction to calculate the sum of two numbers :\n");
Console.Write("--------------------------------------------------\n");
// Prompt the user to enter a number and read the input as an integer
Console.Write("Enter a number: ");
int n1 = Convert.ToInt32(Console.ReadLine());
// Prompt the user to enter another number and read the input as an integer
Console.Write("Enter another number: ");
int n2 = Convert.ToInt32(Console.ReadLine());
// Calculate the sum by calling the 'Sum' method and print the result
Console.WriteLine("\nThe sum of two numbers is : {0} \n", Sum(n1, n2));
}
}
Sample Output:
Function to calculate the sum of two numbers : -------------------------------------------------- Enter a number: 25 Enter another number: 50 The sum of two numbers is : 75
Flowchart :
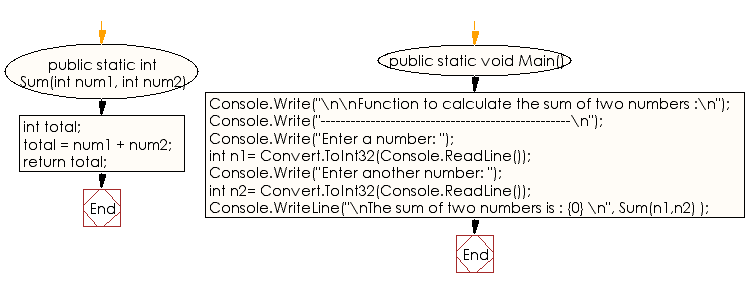
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to create a user define function with parameters.
Next: Write a program in C# Sharp to create a function to input a string and count number of spaces are in the string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.