C#: Learn, how to create a user define function with parameters
C# Sharp Function: Exercise-2 with Solution
Write a program in C# Sharp to create a user defined function with parameters.
Visual Presentation:
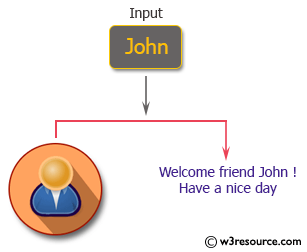
Sample Solution:
C# Sharp Code:
using System;
// Define a public class named 'funcexer2'
public class funcexer2
{
// Define a public static method 'welcome' that takes a string parameter 'name' and prints a welcome message
public static void welcome(string name)
{
Console.WriteLine("Welcome friend " + name + " !");
}
// Define a public static method 'HaveAnice' that prints a message for a nice day
public static void HaveAnice()
{
Console.WriteLine("Have a nice day!");
}
// Main method, the entry point of the program
public static void Main(string[] args)
{
// Print a description of the program
Console.Write("\n\nSee, how to create an user define function with parameters :\n");
Console.Write("----------------------------------------------------------------\n");
// Declare a string variable 'str1'
string str1;
// Ask user for input and read a string from the console
Console.Write("Please input a name : ");
str1 = Console.ReadLine();
// Call the 'welcome' method passing the input string as an argument to print the welcome message
welcome(str1);
// Call the 'HaveAnice' method to print the message for a nice day
HaveAnice();
}
}
Sample Output:
See, how to create an user define function with parameters : ---------------------------------------------------------------- Please input a name : John Welcome friend John ! Have a nice day!
Flowchart :
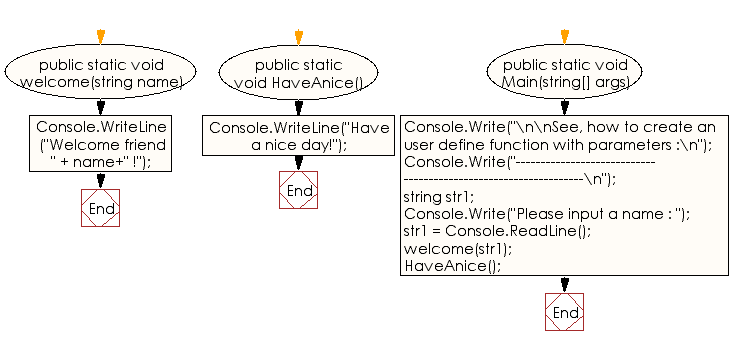
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to create a user define function.
Next: Write a program in C# Sharp to create a function for the sum of two numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/function/csharp-function-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics