C#: To find the factorial of a given number
Write a program in C# Sharp to create a recursive function to find the factorial of a given number.
Visual Presentation:
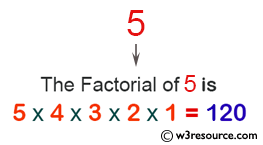
Sample Solution:
C# Sharp Code:
using System;
// Class definition named 'funcexer11'
class funcexer11
{
// Main method, the entry point of the program
static void Main()
{
decimal f;
// Display a description of the program
Console.Write("\n\nRecursive Function : To find the factorial of a given number :\n");
Console.Write("------------------------------------------------------------------\n");
// Prompt the user to input a number
Console.Write("Input a number : ");
int num = Convert.ToInt32(Console.ReadLine());
// Call the 'Factorial' method to calculate the factorial of the input number
f = Factorial(num);
// Display the factorial of the input number
Console.WriteLine("The factorial of {0}! is {1}", num, f);
}
// Recursive method to calculate the factorial of a number
static decimal Factorial(int n1)
{
// The base case of the recursion: factorial of 0 is 1
if (n1 == 0)
{
return 1;
}
// Recursive call: the method calls itself to calculate factorial
else
{
return n1 * Factorial(n1 - 1);
}
}
}
Sample Output:
Recursive Function : To find the factorial of a given number : ------------------------------------------------------------------ Input a number : 5 The factorial of 5! is 120
Flowchart :
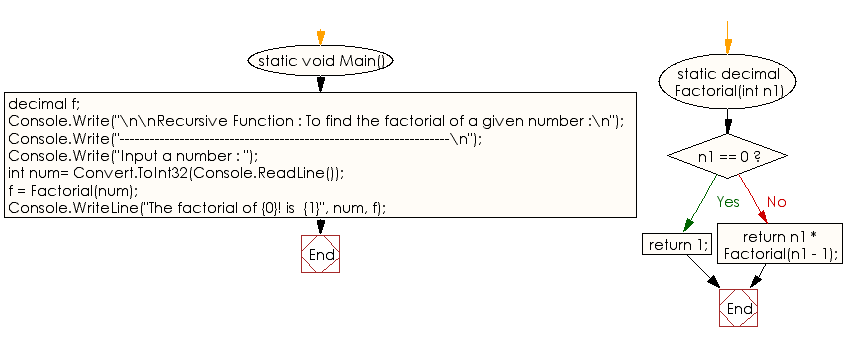
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to create a function to calculate the sum of the individual digits of a given number.
Next: Write a program in C# Sharp to create a recursive function to calculate the Fibonacci number of a specific term.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.