C#: To calculate the sum of the individual digits of a specified number
Write a program in C# Sharp to create a function to calculate the sum of the individual digits of a given number.
Visual Presentation:
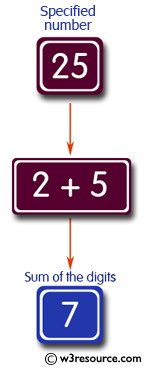
Sample Solution:
C# Sharp Code:
using System;
// Class definition named 'funcexer10'
public class funcexer10
{
// Method to calculate the sum of individual digits of a number
public static int SumCal(int n)
{
// Convert the number to a string
string n1 = Convert.ToString(n);
int sum = 0;
// Loop through each digit in the number
for (int i = 0; i < n1.Length; i++)
{
// Extract each digit and add it to the sum
sum += Convert.ToInt32(n1.Substring(i, 1));
}
return sum; // Return the sum of individual digits
}
// Main method, the entry point of the program
public static void Main()
{
int num;
// Display a description of the program
Console.Write("\n\nFunction : To calculate the sum of the individual digits of a number :\n");
Console.Write("--------------------------------------------------------------------------\n");
// Prompt the user to enter a number
Console.Write("Enter a number: ");
num = Convert.ToInt32(Console.ReadLine());
// Calculate and display the sum of the digits of the input number
Console.WriteLine("The sum of the digits of the number {0} is : {1} \n", num, SumCal(num));
}
}
Sample Output:
Function : To calculate the sum of the individual digits of a number : -------------------------------------------------------------------------- Enter a number: 25 The sum of the digits of the number 25 is : 7
Flowchart :
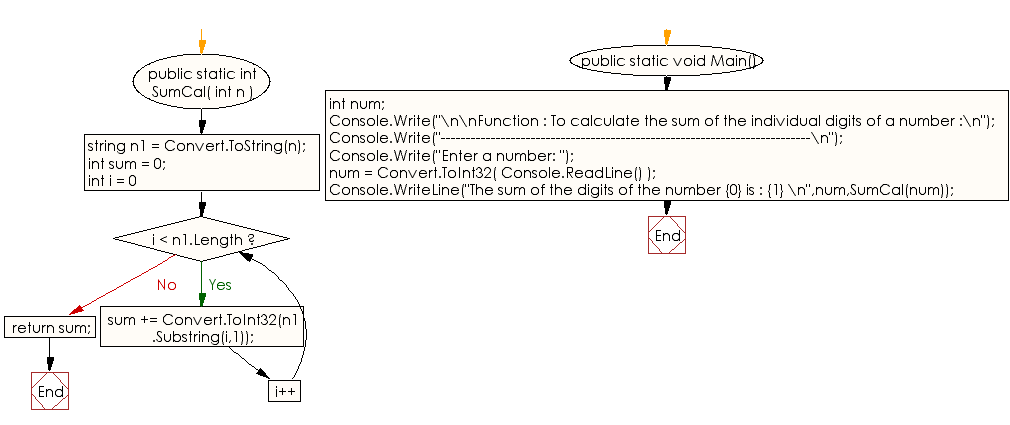
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to create a function to check whether a number is prime or not.
Next: Write a program in C# Sharp to create a recursive function to find the factorial of a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.