C#: Display the pattern like right angle using an asterisk
Write a program in C# Sharp to display a right angle triangle with an asterisk.
Sample Output:
* ** *** ****
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise9 // Declaration of the Exercise9 class
{
public static void Main() // Main method, entry point of the program
{
int i, j, rows; // Declaration of variables 'i', 'j' for iteration, 'rows' to store the number of rows
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the pattern like a right angle using asterisk:\n"); // Displaying the purpose of the program
Console.Write("------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input number of rows : "); // Prompting the user to input the number of rows
rows = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
for (i = 1; i <= rows; i++) // Loop to iterate through each row
{
for (j = 1; j <= i; j++) // Loop to print '*' in each row based on the value of 'i'
{
Console.Write("*"); // Displaying asterisks to form the pattern
}
Console.Write("\n"); // Moving to the next line to form the right-angled pattern
}
}
}
Sample Output:
Display the pattern like right angle using asterisk: ------------------------------------------------------ Input number of rows : 10 * ** *** **** ***** ****** ******* ******** ********* **********
Flowchart:
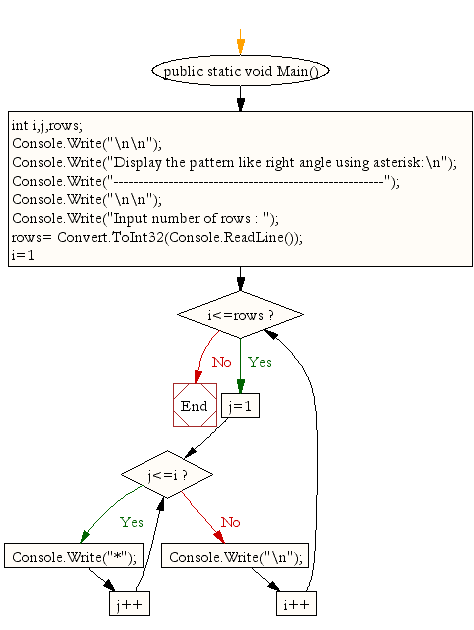
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the n terms of odd natural number and their sum
Next: Write a program in C# Sharp to display following Pattern.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.