C#: Display alphabet pattern like X with an asterisk
Write a C# Sharp program to display an alphabet pattern like X with an asterisk.
Visual Presentation:
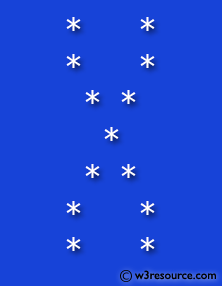
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise81 // Declaration of the Exercise81 class
{
public static void Main() // Main method, entry point of the program
{
int row, column; // Declaring variables for rows and columns
// Display a message about the pattern being created
Console.Write("\n\n");
Console.Write("Display the pattern like 'X' with an asterisk:\n");
Console.Write("---------------------------------------------");
Console.Write("\n\n");
// Loop for iterating through rows
for (row = 0; row <= 6; row++)
{
// Loop for iterating through columns
for (column = 0; column <= 6; column++)
{
// Conditions to determine whether to print '*' or ' ' for specific positions
if (((column == 1 || column == 5) && (row > 4 || row < 2)) || row == column && column > 0 && column < 6 || (column == 2 && row == 4) || (column == 4 && row == 2))
{
Console.Write("*"); // Print '*' for specific conditions
}
else
{
Console.Write(" "); // Print ' ' for other positions
}
}
Console.Write("\n"); // Move to the next line after each row is printed
}
Console.Write("\n"); // Add an extra line at the end for better readability
}
}
Sample Output:
Display the pattern like 'X' with an asterisk: --------------------------------------------- * * * * * * * * * * * * *
Flowchart:
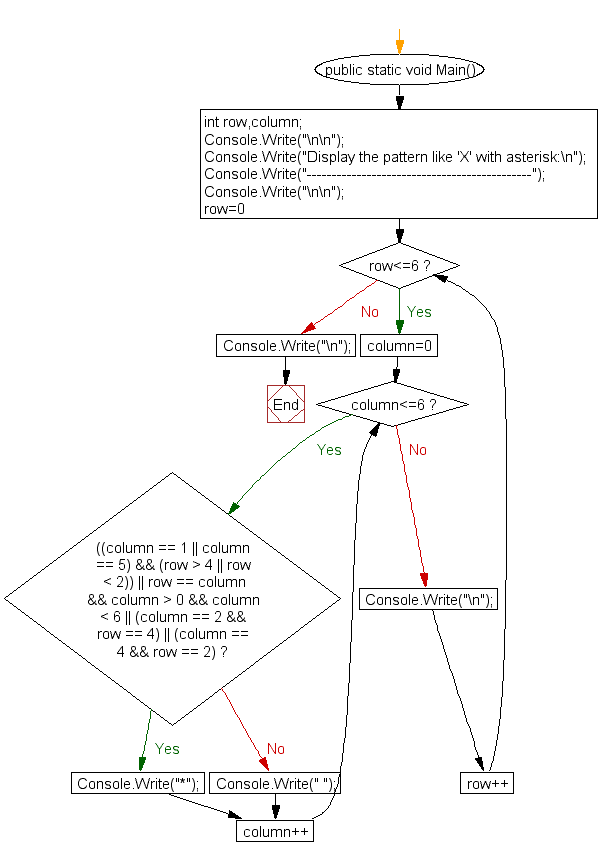
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C#Sharp program to display alphabet pattern like 'W' with an asterisk.
Next: Write a C#Sharp program to display alphabet pattern like 'Y' with an asterisk.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.