C#: Display n number of multiplication table vertically
C# Sharp For Loop: Exercise-7 with Solution
Write a program in C# Sharp to display the multiplication table vertically from 1 to n.
Visual Presentation:
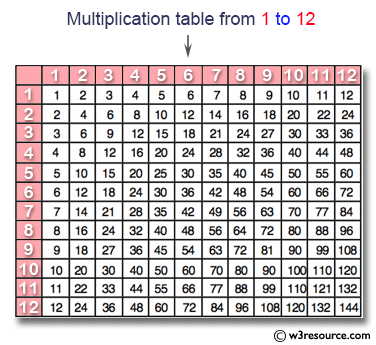
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise7 // Declaration of the Exercise7 class
{
public static void Main() // Main method, entry point of the program
{
int j, i, n; // Declaration of variables 'j' and 'i' for iteration and 'n' to store the input number
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the multiplication table vertically from 1 to n:\n"); // Displaying the purpose of the program
Console.Write("---------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input up to the table number starting from 1 : "); // Prompting the user to input a number for the multiplication table
n = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
Console.Write("Multiplication table from 1 to {0} \n", n); // Displaying the range of multiplication table
for (i = 1; i <= 10; i++) // Outer loop to generate rows for the multiplication table up to 10
{
for (j = 1; j <= n; j++) // Inner loop to generate columns for each number up to 'n'
{
if (j <= n - 1) // Checking if it's not the last column of the table
Console.Write("{0}x{1} = {2}, ", j, i, i * j); // Displaying the multiplication table entry with comma separation
else
Console.Write("{0}x{1} = {2}", j, i, i * j); // Displaying the multiplication table entry for the last column
}
Console.Write("\n"); // Moving to the next line after completing a row in the table
}
}
}
Sample Output:
Display the multiplication table vertically from 1 to n: --------------------------------------------------------- Input upto the table number starting from 1 : 5 Multiplication table from 1 to 5 1x1 = 1, 2x1 = 2, 3x1 = 3, 4x1 = 4, 5x1 = 5 1x2 = 2, 2x2 = 4, 3x2 = 6, 4x2 = 8, 5x2 = 10 1x3 = 3, 2x3 = 6, 3x3 = 9, 4x3 = 12, 5x3 = 15 1x4 = 4, 2x4 = 8, 3x4 = 12, 4x4 = 16, 5x4 = 20 1x5 = 5, 2x5 = 10, 3x5 = 15, 4x5 = 20, 5x5 = 25 1x6 = 6, 2x6 = 12, 3x6 = 18, 4x6 = 24, 5x6 = 30 1x7 = 7, 2x7 = 14, 3x7 = 21, 4x7 = 28, 5x7 = 35 1x8 = 8, 2x8 = 16, 3x8 = 24, 4x8 = 32, 5x8 = 40 1x9 = 9, 2x9 = 18, 3x9 = 27, 4x9 = 36, 5x9 = 45 1x10 = 10, 2x10 = 20, 3x10 = 30, 4x10 = 40, 5x10 = 50
Flowchart:
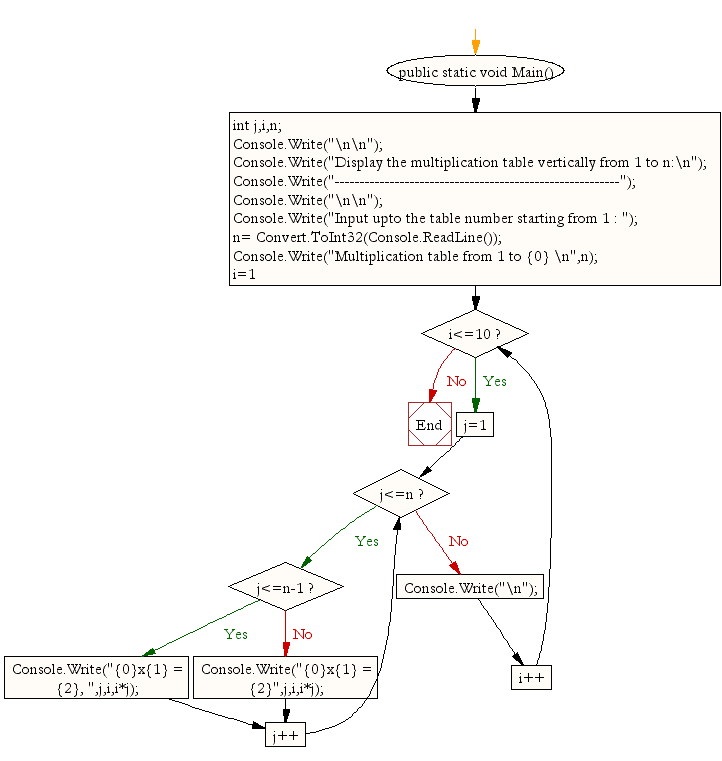
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the multiplication table of a given integer.
Next: Write a program in C# Sharp to display the n terms of odd natural number and their sum.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/for-loop/csharp-for-loop-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics