C#: Convert Octal number to Decimal number
Write a program in C# Sharp to convert an octal number to decimal without using an array.
Visual Presentation:
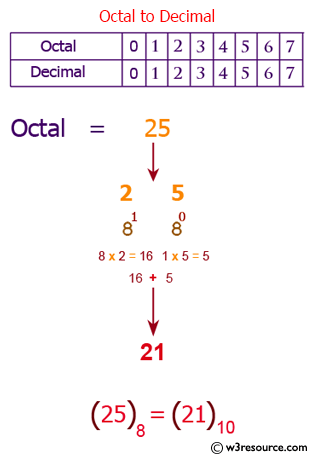
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise51 // Declaration of the Exercise51 class
{
public static void Main() // Main method, entry point of the program
{
// Declaration of variables
int n1, n5, p = 1, k, ch = 1;
int dec = 0, i = 1, j, d;
// Displaying information about converting octal to decimal
Console.Write("\n\n");
Console.Write("Convert Octal number to Decimal number:\n");
Console.Write("------------------------------------------");
Console.Write("\n\n");
// Prompting the user to enter an octal number
Console.Write("Input an octal number (using digit 0 - 7) :");
n1 = Convert.ToInt32(Console.ReadLine()); // Reading the octal number
n5 = n1; // Storing the original number for display
// Loop to check if the entered number is a valid octal number
for (; n1 > 0; n1 = n1 / 10)
{
k = n1 % 10;
if (k >= 8)
{
ch = 0; // If a digit is more than 7, it's not a valid octal number
}
}
// Switch statement to handle validation and conversion
switch (ch)
{
case 0:
Console.Write("\nThe number is not an octal number. \n\n");
break;
case 1:
n1 = n5; // Restoring the original octal number
// Loop to convert octal to decimal
for (j = n1; j > 0; j = j / 10)
{
d = j % 10;
if (i == 1)
p = p * 1;
else
p = p * 8;
dec = dec + (d * p);
i++;
}
// Displaying the converted decimal number
Console.Write("\nThe Octal Number : {0}\nThe equivalent Decimal Number : {1} \n\n", n5, dec);
break;
}
}
}
Sample Output:
Convert Octal number to Decimal number: ------------------------------------------ Input an octal number (using digit 0 - 7) :5 The Octal Number : 5 The equivalent Decimal Number : 5
Flowchart:
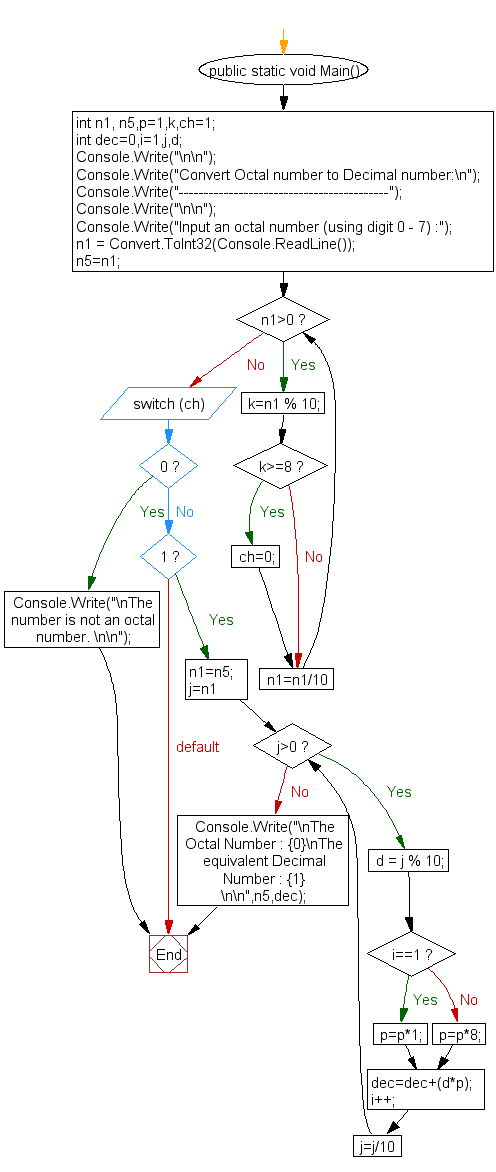
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to convert a decimal number into octal without an using an array.
Next: Write a program in C# Sharp to find the Sum of GP series.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.