C#: Find cube of the number up to given an integer
Write a C# Sharp program to display the cube of an integer up to given number.
Visual Presentation:
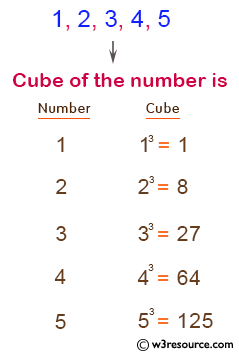
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise5 // Declaration of the Exercise5 class
{
public static void Main() // Main method, entry point of the program
{
int i, ctr; // Declaration of variables 'i' for iteration and 'ctr' to store the number of terms
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the cube of the number:\n"); // Displaying the purpose of the program
Console.Write("---------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input number of terms : "); // Prompting the user to input the number of terms
ctr = Convert.ToInt32(Console.ReadLine()); // Reading the number of terms entered by the user
for (i = 1; i <= ctr; i++) // Loop to calculate and display the cube of each number from 1 to 'ctr'
{
Console.Write("Number is : {0} and cube of the {1} is :{2} \n", i, i, (i * i * i)); // Displaying the number and its cube
}
}
}
Sample Output:
Display the cube of the number: --------------------------------- Input number of terms : 5 Number is : 1 and cube of the 1 is :1 Number is : 2 and cube of the 2 is :8 Number is : 3 and cube of the 3 is :27 Number is : 4 and cube of the 4 is :64 Number is : 5 and cube of the 5 is :125
Flowchart:
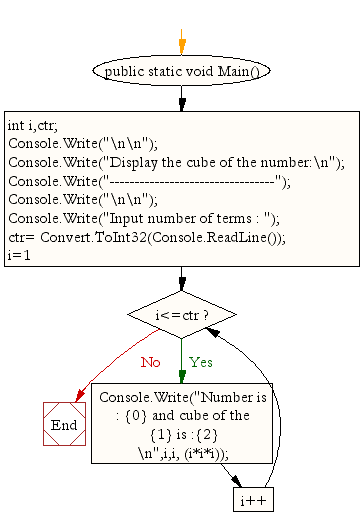
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to read 10 numbers from keyboard and find their sum and average.
Next: Write a program in C# Sharp to display the multiplication table of a given integer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.