C#: Convert a binary to a decimal using for loop and without using an array
Write a program in C# Sharp to convert a binary number into a decimal number without using an array, function and while loop.
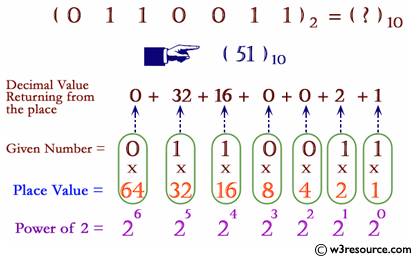
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise42 // Declaration of the Exercise42 class
{
public static void Main() // Main method, entry point of the program
{
int n1, n, p = 1; // Declaration of variables
int dec = 0, i = 1, j, d; // Initialization of variables for decimal conversion
Console.Write("\n\n"); // Displaying new lines
Console.Write("Convert a binary to decimal using for loop and without using array:\n"); // Displaying the purpose of the program
Console.Write("---------------------------------------------------------------------\n\n"); // Displaying a separator and new lines
Console.Write("Input a binary number :"); // Prompting user to input a binary number
n = Convert.ToInt32(Console.ReadLine()); // Reading user input
n1 = n; // Storing the original binary number for display
for (j = n; j > 0; j = j / 10) // Loop to convert binary to decimal
{
d = j % 10; // Extracting each digit from the binary number
if (i == 1)
p *= 1; // Power value for the first digit is 1
else
p *= 2; // Power value for subsequent digits is doubled
dec = dec + (d * p); // Calculating decimal equivalent by multiplying digit by corresponding power of 2
i++; // Incrementing the power index for each digit position
}
Console.Write("\nThe Binary Number : {0}\nThe equivalent Decimal Number : {1} \n\n", n1, dec); // Displaying the converted decimal number
}
}
Sample Output:
Convert a binary to decimal using for loop and without using array: --------------------------------------------------------------------- Input a binary number :1000001 The Binary Number : 1000001 The equivalent Decimal Number : 65
Flowchart:
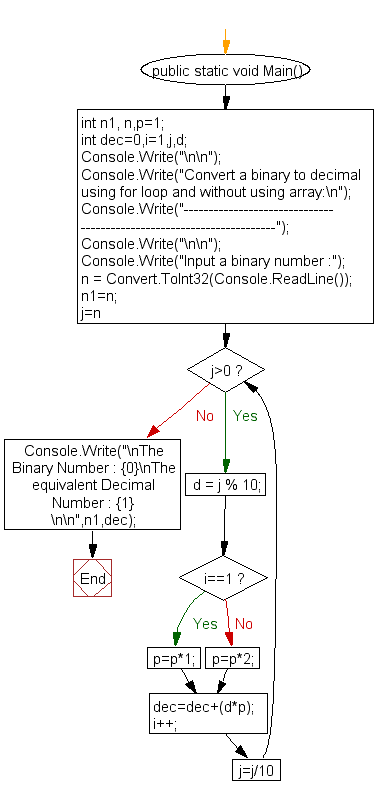
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to convert a decimal number into binary without using an array.
Next: Write a C# Sharp program to find HCF (Highest Common Factor) of two numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.