C#: Convert a decimal number to binary without using an array
Write a program in C# Sharp to convert a decimal number into binary without using an array.
Visual Presentation:
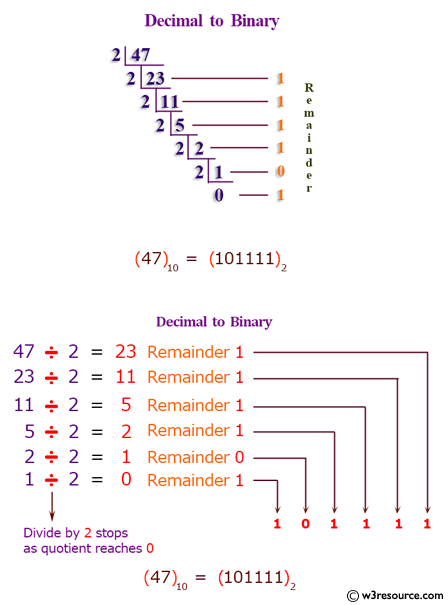
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise41 // Declaration of the Exercise41 class
{
public static void Main() // Main method, entry point of the program
{
int n, i, j, binno = 0, dn; // Declaration of variables
Console.Write("\n\n"); // Displaying new lines
Console.Write("Convert a decimal number to binary without using array:\n"); // Displaying the purpose of the program
Console.Write("---------------------------------------------------------\n\n"); // Displaying a separator and new lines
Console.Write("Enter a number to convert : "); // Prompting user to enter a number
n = Convert.ToInt32(Console.ReadLine()); // Reading user input
dn = n; // Storing the original decimal number
i = 1; // Initializing variable for binary conversion
for (j = n; j > 0; j = j / 2) // Loop to convert decimal to binary
{
binno = binno + (n % 2) * i; // Calculating binary digits
i = i * 10; // Shifting position for next binary digit
n = n / 2; // Updating the decimal number for next iteration
}
Console.Write("\nThe Binary of {0} is {1}.\n\n", dn, binno); // Displaying the converted binary number
}
}
Sample Output:
Convert a decimal number to binary without using array: --------------------------------------------------------- Enter a number to convert : 65 The Binary of 65 is 1000001.
Flowchart:
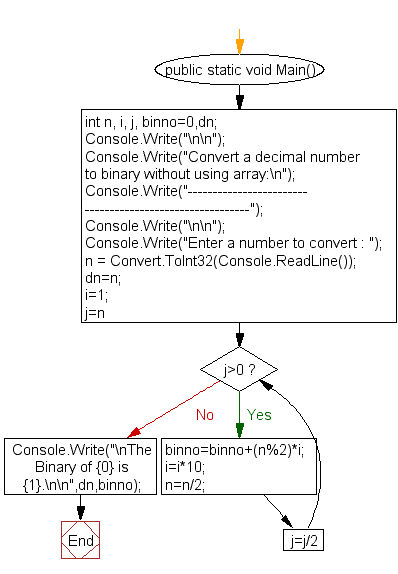
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp Program to display the following pattern using the alphabet.
Next: Write a program in C# Sharp to convert a binary number into a decimal number without using an array, function and while loop.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.