C#: Display the pattern like pyramid using the alphabet
Write a C# Sharp Program to display the following pattern using the alphabet.
The pattern is as follows:
A A B A A B C B A A B C D C B A
Visual Presentation:
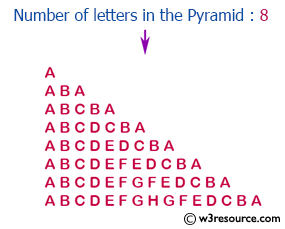
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise40 // Declaration of the Exercise40 class
{
public static void Main() // Main method, entry point of the program
{
int i, j; // Declaration of loop control variables
char alph = 'A'; // Variable to store alphabets
int n; // Variable to store the number of letters in the pyramid
int ctr = 1; // Counter variable for pyramid construction
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the pattern like pyramid using the alphabet:\n"); // Displaying the purpose of the program
Console.Write("------------------------------------------------------\n\n"); // Displaying a separator and new lines
Console.Write("Input the number of Letters (less than 26) in the Pyramid : \n"); // Prompting user to input the number of letters
n = Convert.ToInt32(Console.ReadLine()); // Reading user input for the number of letters in the pyramid
for (i = 1; i <= n; i++) // Outer loop for the number of rows in the pyramid
{
for (j = 0; j <= (ctr / 2); j++) // Loop to print the first half of letters in each row
{
Console.Write("{0} ", alph++); // Printing the letters in ascending order
}
alph--; // Decrementing the alphabet to start printing the second half
for (j = 0; j < (ctr / 2); j++) // Loop to print the second half of letters in each row
{
Console.Write("{0} ", alph--); // Printing the letters in descending order
}
ctr = ctr + 2; // Increasing the counter for the next row
alph = 'A'; // Resetting the alphabet to 'A' for the next row
Console.Write("\n"); // Moving to the next line for the new row
}
}
}
Sample Output:
Display the pattern like pyramid using the alphabet: ------------------------------------------------------ Input the number of Letters (less than 26) in the Pyramid : 8 A A B A A B C B A A B C D C B A A B C D E D C B A A B C D E F E D C B A A B C D E F G F E D C B A A B C D E F G H G F E D C B A
Flowchart:
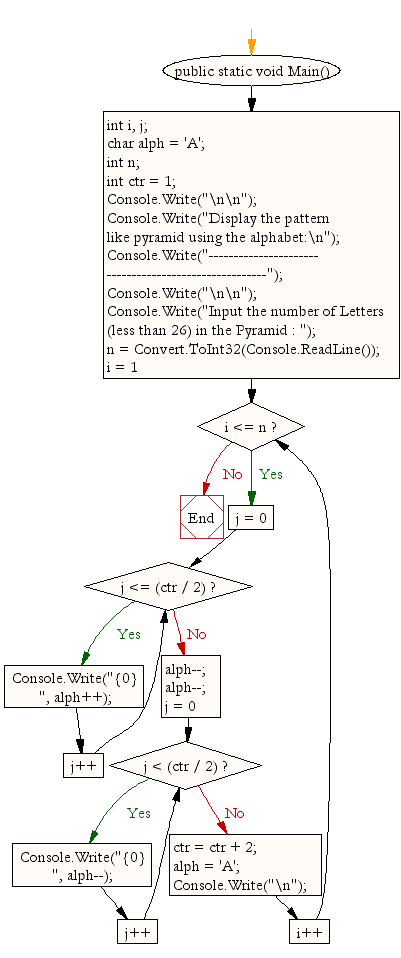
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the number and sum of all integer between 100 and 200 which are divisible by 9.
Next: Write a program in C# Sharp to convert a decimal number into binary without using an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics