C#: Display the pattern in which the first and a last number of each row will be 1
Write a program in C# Sharp to display such a pattern for n number of rows using a number starting with the number 1. The first and last number of each row will be 1..
The pattern is as follows :
1 121 12321
Visual Presentation:
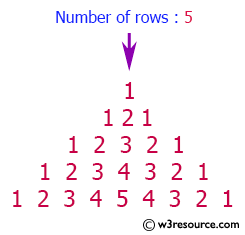
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise36 // Declaration of the Exercise36 class
{
public static void Main() // Main method, entry point of the program
{
int i, j, n; // Declaration of variables for row and column iteration
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the pattern in which the first and last number of each row will be 1:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------------------------------------\n\n"); // Displaying a separator and new lines
Console.Write("Input number of rows: "); // Prompting the user to input the number of rows
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of rows entered by the user
for (i = 0; i <= n; i++) // Loop for generating rows
{
/* print blank spaces */
for (j = 1; j <= n - i; j++) // Loop to print leading spaces in each row
Console.Write(" ");
/* Display numbers in ascending order up to the middle */
for (j = 1; j <= i; j++) // Loop to print numbers in ascending order
Console.Write("{0}", j);
/* Display numbers in reverse order after reaching the middle */
for (j = i - 1; j >= 1; j--) // Loop to print numbers in reverse order
Console.Write("{0}", j);
Console.Write("\n"); // Move to the next line for the next row
}
}
}
Sample Output:
Display the pattern in which first and last number of each row will be 1: ---------------------------------------------------------------------------- Input number of rows : 5 1 121 12321 1234321 123454321
Flowchart:
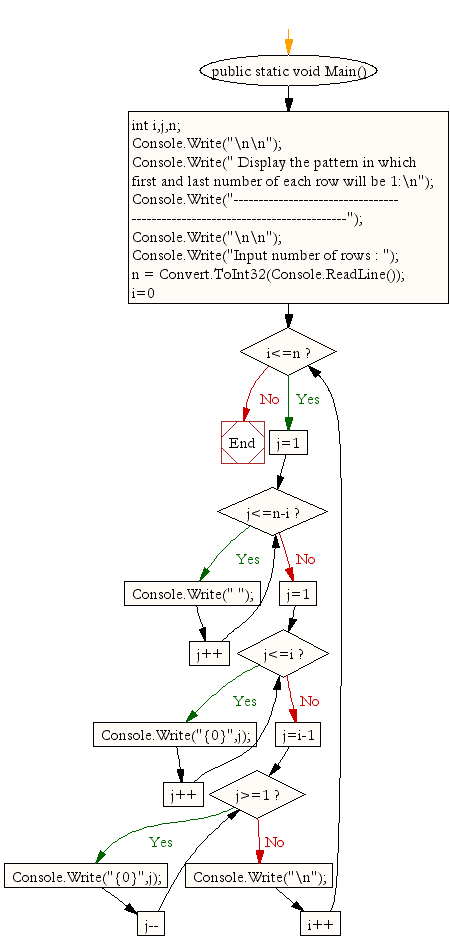
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to display the first n terms of Fibonacci series.
Next: Write a program in C# Sharp to display the number in reverse order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.