C#: Check whether a given number is prime or not
Write a C# Sharp Program to determine whether a given number is prime or not.
Visual Presentation:
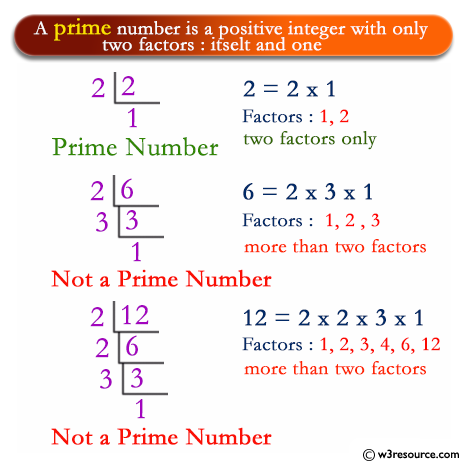
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise32 // Declaration of the Exercise32 class
{
public static void Main() // Main method, entry point of the program
{
int num, i, ctr = 0; // Declaration of variables num, i, and ctr as integers
Console.Write("\n\n"); // Displaying new lines
Console.Write("Check whether a given number is prime or not:\n"); // Displaying the purpose of the program
Console.Write("-----------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Displaying new lines
Console.Write("Input a number: "); // Prompting the user to input a number
num = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
// Loop to check if the number is prime
for (i = 2; i <= num / 2; i++)
{
if (num % i == 0)
{
ctr++; // Incrementing the counter if the number has divisors other than 1 and itself
break; // Exiting the loop if a divisor is found
}
}
// Checking the value of ctr to determine if the number is prime or not
if (ctr == 0 && num != 1)
{
Console.Write("{0} is a prime number.\n", num); // Displaying if the number is prime
}
else
{
Console.Write("{0} is not a prime number\n", num); // Displaying if the number is not prime
}
}
}
Sample Output:
Check whether a given number is prime or not: ----------------------------------------------- Input a number: 53 53 is a prime number.
Flowchart:
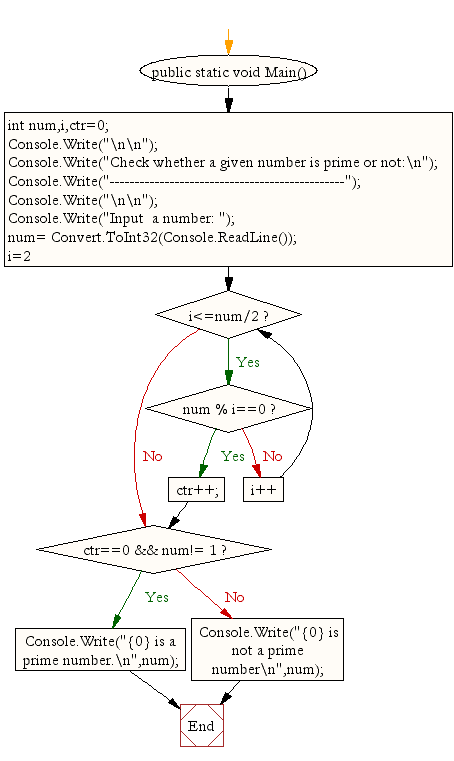
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C to display the pattern like a diamond.
Next: Write a C# Sharp Program to display by Pascal's triangle
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics