C#: Display the pattern like a diamond
C# Sharp For Loop: Exercise-31 with Solution
Write a program in C to display the pattern like a diamond.
The pattern is as follows :
* *** ***** ******* ********* ******* ***** *** *
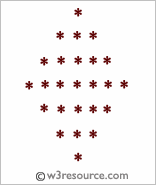
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise31 // Declaration of the Exercise31 class
{
public static void Main() // Main method, entry point of the program
{
int i, j, r; // Declaration of variables i, j, and r as integers
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the pattern like a diamond:\n"); // Displaying the purpose of the program
Console.Write("-----------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Displaying new lines
Console.Write("Input the number of rows (half of the diamond): "); // Prompting the user to input the number of rows
r = Convert.ToInt32(Console.ReadLine()); // Reading the number of rows entered by the user
// Loop to print the upper part of the diamond pattern
for (i = 0; i <= r; i++)
{
for (j = 1; j <= r - i; j++)
Console.Write(" "); // Printing spaces before the asterisks
for (j = 1; j <= 2 * i - 1; j++)
Console.Write("*"); // Printing asterisks to form the upper part of the diamond
Console.Write("\n"); // Moving to the next line after each row
}
// Loop to print the lower part of the diamond pattern
for (i = r - 1; i >= 1; i--)
{
for (j = 1; j <= r - i; j++)
Console.Write(" "); // Printing spaces before the asterisks
for (j = 1; j <= 2 * i - 1; j++)
Console.Write("*"); // Printing asterisks to form the lower part of the diamond
Console.Write("\n"); // Moving to the next line after each row
}
}
}
Sample Output:
Display the pattern like diamond: ----------------------------------- Input number of rows (half of the diamond) :8 * *** ***** ******* ********* *********** ************* *************** ************* *********** ********* ******* ***** *** *
Flowchart:
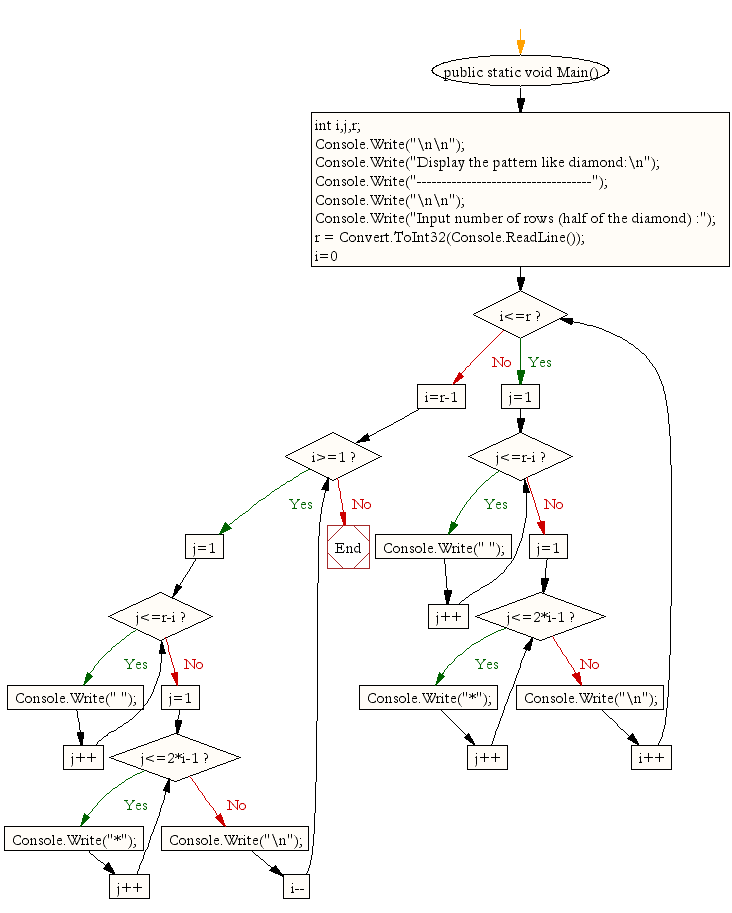
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp Program to find the Armstrong number for a given range of number.
Next: Write a C# Sharp Program to determine whether a given number is prime or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/for-loop/csharp-for-loop-exercise-31.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics