C#: Find the Armstrong number for a given range of number
Write a C# Sharp program to find the Armstrong number for a given range of numbers.
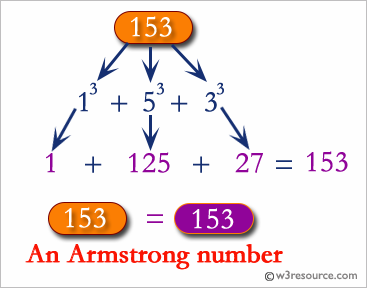
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise30 // Declaration of the Exercise30 class
{
public static void Main() // Main method, entry point of the program
{
int num, r, sum, temp; // Declaration of variables num, r, sum, and temp as integers
int stno, enno; // Declaration of variables stno and enno as integers
Console.Write("\n\n"); // Displaying new lines
Console.Write("Find the Armstrong numbers for a given range of numbers:\n"); // Displaying the purpose of the program
Console.Write("--------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Displaying new lines
Console.Write("Input the starting number of the range: "); // Prompting the user to input the starting number of the range
stno = Convert.ToInt32(Console.ReadLine()); // Reading the starting number of the range entered by the user
Console.Write("Input the ending number of the range: "); // Prompting the user to input the ending number of the range
enno = Convert.ToInt32(Console.ReadLine()); // Reading the ending number of the range entered by the user
Console.Write("Armstrong numbers in the given range are: "); // Displaying a message to indicate the output
// Loop to iterate through numbers within the given range
for (num = stno; num <= enno; num++)
{
temp = num; // Storing the original number in the 'temp' variable
sum = 0; // Initializing the sum variable to 0
// Loop to calculate the sum of cubes of individual digits of the number
while (temp != 0)
{
r = temp % 10; // Extracting the rightmost digit of the number
temp = temp / 10; // Removing the rightmost digit from the number
sum = sum + (r * r * r); // Calculating the sum of cubes of digits
}
// Checking if the calculated sum of cubes of digits is equal to the original number
if (sum == num)
Console.Write("{0} ", num); // Displaying the number if it's an Armstrong number within the range
}
Console.Write("\n"); // Displaying a new line at the end
}
}
Sample Output:
Find the Armstrong number for a given range of number: -------------------------------------------------------- Input starting number of range: 1 Input ending number of range : 500 Armstrong numbers in given range are: 1 153 370 371 407
Flowchart:
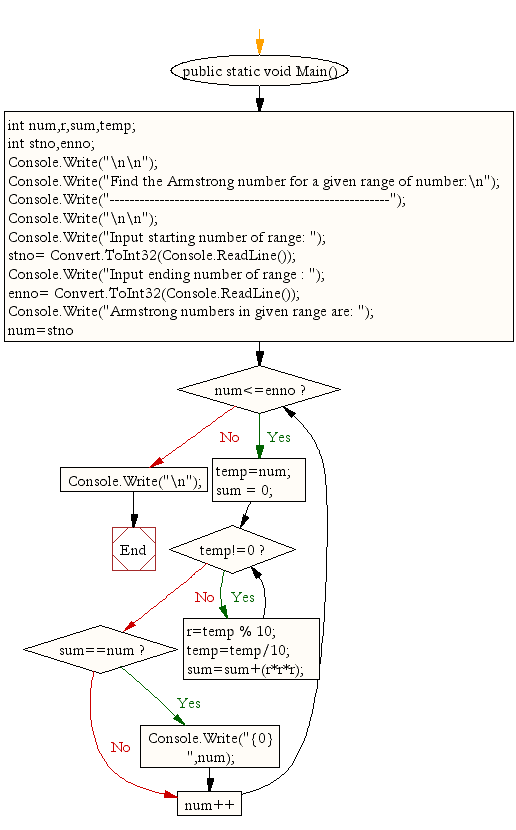
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp Program to check whether a given number is an Armstrong number or not.
Next: Write a program in C to display the pattern like a diamond.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.