C#: Display n natural numbers and their sum
Write a C# Sharp program that displays the sum of n natural numbers.
Visual Presentation:
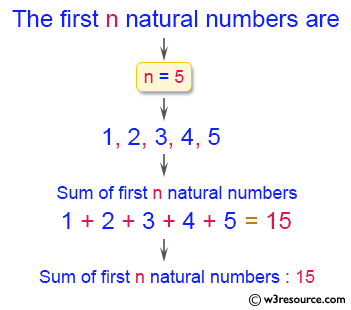
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise3 // Declaration of the Exercise3 class
{
public static void Main() // Main method, entry point of the program
{
int i, n, sum = 0; // Declaration of variables 'i' for iteration, 'n' for storing user input, and 'sum' to store the total sum
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display n terms of natural numbers and their sum:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input Value of terms : "); // Prompting the user to input the number of terms
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of terms entered by the user
Console.Write("\nThe first {0} natural numbers are:\n", n); // Displaying the header for the natural numbers
for (i = 1; i <= n; i++) // Loop to display and calculate the sum of the first 'n' natural numbers
{
Console.Write("{0} ", i); // Printing each natural number
sum += i; // Adding each number to the sum
}
Console.Write("\nThe Sum of Natural Numbers up to {0} terms : {1} \n", n, sum); // Displaying the total sum of the first 'n' natural numbers
}
}
Sample Output:
Display n terms of natural number and their sum: ---------------------------------------------- Input Value of terms : 5 The first 5 natural number are : 1 2 3 4 5 The Sum of Natural Number upto 5 terms : 15
Flowchart:
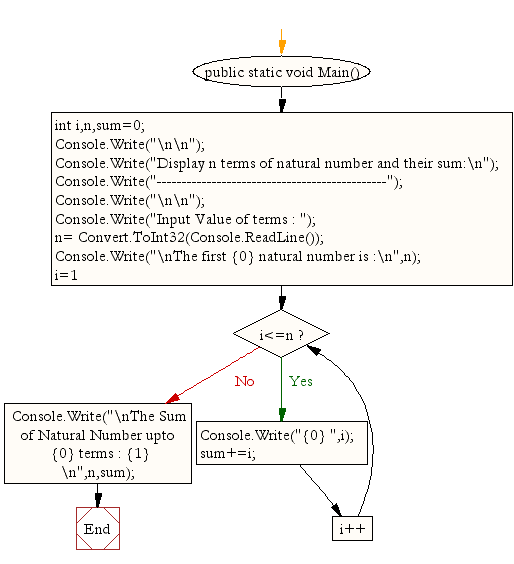
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp program to find the sum of first 10 natural numbers.
Next: Write a program in C# Sharp to read 10 numbers from keyboard and find their sum and average.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics