C#: Check whether a given number is an Armstrong number or not
Write a C# Sharp Program to check whether a given number is an Armstrong number or not.
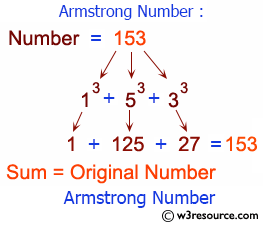
Sample Solution:-
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise29 // Declaration of the Exercise29 class
{
public static void Main() // Main method, entry point of the program
{
int num, r, sum = 0, temp; // Declaration of variables num, r, sum, and temp as integers
Console.Write("\n\n"); // Displaying new lines
Console.Write("Check whether a given number is an Armstrong number or not:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Displaying new lines
Console.Write("Input a number: "); // Prompting the user to input a number
num = Convert.ToInt32(Console.ReadLine()); // Reading the number entered by the user
temp = num; // Storing the original number in the 'temp' variable
// Loop to calculate the sum of cubes of individual digits of the number
for (; num != 0; num = num / 10)
{
r = num % 10; // Extracting the rightmost digit of the number
sum = sum + (r * r * r); // Calculating the sum of cubes of digits
}
// Checking if the calculated sum of cubes of digits is equal to the original number
if (sum == temp)
Console.Write("{0} is an Armstrong number.\n", temp); // Displaying the result if it's an Armstrong number
else
Console.Write("{0} is not an Armstrong number.\n", temp); // Displaying the result if it's not an Armstrong number
}
}
Sample Output:
Check whether a given number is armstrong number or not: ---------------------------------------------------------- Input a number: 153 153 is an Armstrong number.
Flowchart:
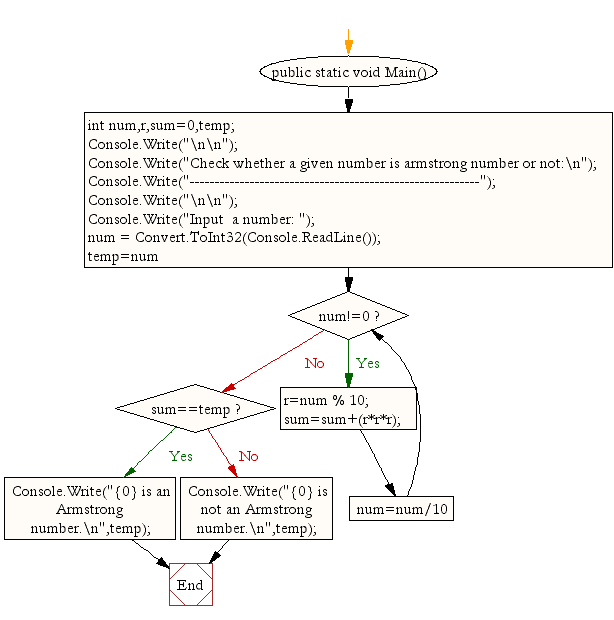
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp Program to find the perfect numbers within a given range of number.
Next: Write a C# Sharp Program to find the Armstrong number for a given range of number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics