C#: Find perfect numbers within a given range of number
C# Sharp For Loop: Exercise-28 with Solution
Write a C# Sharp program to find the ‘perfect’ numbers within a given number range.
Visual Presentation:
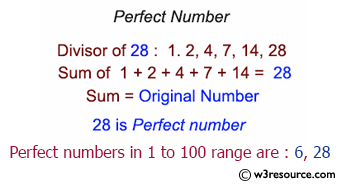
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise28 // Declaration of the Exercise28 class
{
public static void Main() // Main method, entry point of the program
{
int n, i, sum; // Declaration of variables n, i, and sum as integers
int mn, mx; // Declaration of variables mn and mx as integers
Console.Write("\n\n"); // Displaying new lines
Console.Write("Find perfect numbers within a given range:\n"); // Displaying the purpose of the program
Console.Write("------------------------------------------------------"); // Displaying a separator
Console.Write("\n\n"); // Displaying new lines
Console.Write("Input the starting range or number : "); // Prompting the user to input the starting range
mn = Convert.ToInt32(Console.ReadLine()); // Reading the starting range entered by the user
Console.Write("Input the ending range of number : "); // Prompting the user to input the ending range
mx = Convert.ToInt32(Console.ReadLine()); // Reading the ending range entered by the user
Console.Write("The Perfect numbers within the given range : "); // Displaying a message
// Loop to find perfect numbers within the given range
for (n = mn; n <= mx; n++)
{
i = 1; // Initializing i to 1
sum = 0; // Initializing sum to 0
// Loop to calculate the sum of divisors for each number in the range
while (i < n)
{
if (n % i == 0) // Checking if 'i' is a divisor of 'n'
sum = sum + i; // Calculating the sum of divisors
i++; // Incrementing 'i'
}
// Checking if the sum of divisors is equal to the original number
if (sum == n)
Console.Write("{0} ", n); // Displaying the perfect number
}
Console.Write("\n"); // Displaying a new line
}
}
Sample Output:
Find perfect numbers within a given number of range: ------------------------------------------------------ Input the starting range or number : 1 Input the ending range of number : 20 The Perfect numbers within the given range : 6
Flowchart:
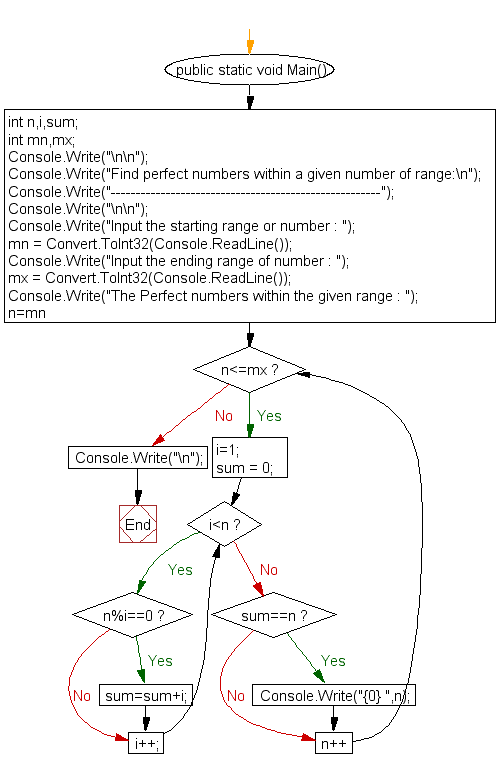
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a C# Sharp Program to check whether a given number is perfect number or not.
Next: Write a C# Sharp Program to check whether a given number is an Armstrong number or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/for-loop/csharp-for-loop-exercise-28.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics