C#: Calculate the harmonic series and their sum
Write a program in C# Sharp to display the n terms of harmonic series and their sum. The series is :
1 + 1/2 + 1/3 + 1/4 + 1/5 ... 1/n terms
Visual Presentation:
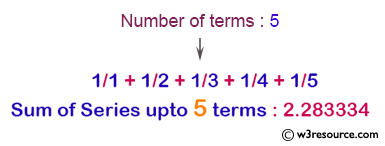
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise19 // Declaration of the Exercise19 class
{
public static void Main() // Main method, entry point of the program
{
int i, n; // Declaration of variables of type integer
double s = 0.0; // Declaration of a variable of type double, initialized to 0.0
Console.Write("\n\n"); // Displaying new lines
Console.Write("Calculate the harmonic series and their sum:\n"); // Displaying the purpose of the program
Console.Write("----------------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.Write("Input the number of terms : "); // Prompting the user to input the number of terms
n = Convert.ToInt32(Console.ReadLine()); // Reading the number of terms entered by the user
Console.Write("\n\n");
// Loop to display and calculate the sum of the harmonic series
for (i = 1; i <= n; i++)
{
Console.Write("1/{0} + ", i); // Displaying the term of the harmonic series
s += 1 / (float)i; // Calculating and adding the current term to the sum
}
Console.Write("\nSum of Series upto {0} terms : {1} \n", n, s); // Displaying the sum of the series
}
}
Sample Output:
Calculate the harmonic series and their sum: ---------------------------------------------- Input the number of terms : 6 1/1 + 1/2 + 1/3 + 1/4 + 1/5 + 1/6 + Sum of Series upto 6 terms : 2.45
Flowchart:
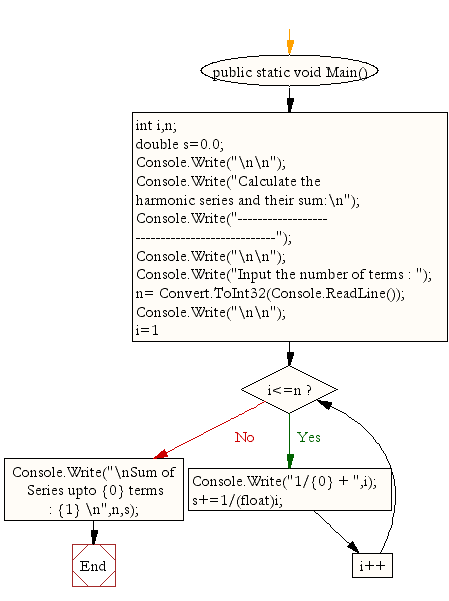
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to find the sum of the series [ 1-X^2/2!+X^4/4!- .........].
Next: Write a program in C# Sharp to display the pattern like a pyramid using asterisk and each row contain an odd number of asterisks.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.