C#: Display first 10 natural numbers
Write a program in C# Sharp to display the first 10 natural numbers.
Visual Presentation:
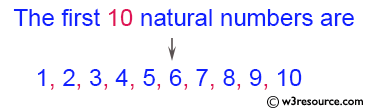
Sample Solution:
C# Sharp Code:
using System; // Importing necessary namespace
public class Exercise1 // Declaration of the Exercise1 class
{
public static void Main() // Main method, entry point of the program
{
int i; // Declaration of variable 'i' to store natural numbers
Console.Write("\n\n"); // Displaying new lines
Console.Write("Display the first 10 natural numbers:\n"); // Displaying the purpose of the program
Console.Write("---------------------------------------"); // Displaying a separator
Console.Write("\n\n");
Console.WriteLine("The first 10 natural numbers are:"); // Displaying a message for the user
for (i = 1; i <= 10; i++) // Loop to print the first 10 natural numbers
{
Console.Write("{0} ", i); // Printing each natural number
}
Console.Write("\n\n"); // Displaying new lines
}
}
Sample Output:
Display the first 10 natural numbers: --------------------------------------- The first 10 natural number are: 1 2 3 4 5 6 7 8 9 10
Flowchart:
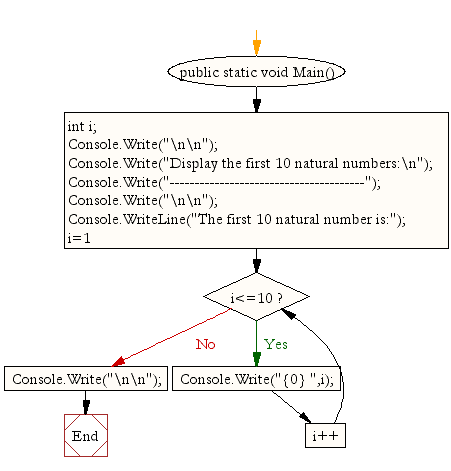
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: C# Sharp programming exercises: For Loop
Next: Write a C# Sharp program to find the sum of first 10 natural numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.