C#: Read last n number of lines from a file
Write a C# Sharp program to create and read the last n lines of a file.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace for basic functionalities
using System.IO; // Importing the System.IO namespace for file operations
class filexercise14 // Declaring a class named filexercise14
{
static void Main() // Declaring the Main method
{
string fileName = @"mytest.txt"; // Initializing a string variable with the file name
string[] ArrLines; // Declaring an array to store lines of text
int n, i, l, m = 1; // Declaring variables for iteration, line count, specific line to read, and iteration counter
// Displaying a message to read the last n number of lines from a file
Console.Write("\n\n Read last n number of lines from a file :\n");
Console.Write("-----------------------------------------------\n");
if (File.Exists(fileName)) // Checking if the file exists and deleting it if true
{
File.Delete(fileName); // Deleting the file if it exists
}
Console.Write(" Input number of lines to write in the file :");
n = Convert.ToInt32(Console.ReadLine()); // Reading user input for the number of lines
ArrLines = new string[n]; // Initializing the array to store lines
Console.Write(" Input {0} strings below :\n", n); // Prompting user to input lines
for (i = 0; i < n; i++)
{
Console.Write(" Input line {0} : ", i + 1);
ArrLines[i] = Console.ReadLine(); // Reading lines of text from user input
}
// Writing all lines to the file
System.IO.File.WriteAllLines(fileName, ArrLines);
Console.Write("\n Input last how many numbers of lines you want to display :");
l = Convert.ToInt32(Console.ReadLine()); // Reading user input for the number of last lines to display
m = l; // Storing l in m for iteration purposes
if (l >= 1 && l <= n) // Checking if the specified number of lines is within the valid range
{
Console.Write("\n The content of the last {0} lines of the file {1} is : \n", l, fileName);
if (File.Exists(fileName)) // Checking if the file exists
{
for (i = n - l; i < n; i++) // Loop to read and display last 'l' lines
{
string[] lines = File.ReadAllLines(fileName); // Reading all lines from the file
Console.Write(" The last no {0} line is : {1} \n", m, lines[i]); // Writing specific lines to the console
m--; // Decrementing m for the next line number display
}
}
}
else
{
Console.WriteLine(" Please input the correct number of lines."); // Displaying message for incorrect line number input
}
Console.WriteLine();
}
}
Sample Output:
Read last n number of lines from a file : ----------------------------------------------- Input number of lines to write in the file :4 Input 4 strings below : Input line 1 : 1st line Input line 2 : 2nd line Input line 3 : 3rd line Input line 4 : 4th line Input last how many numbers of lines you want to display :2 The content of the last 2 lines of the file mytest.txt is : The last no 2 line is : 3rd line The last no 1 line is : 4th line
Flowchart :
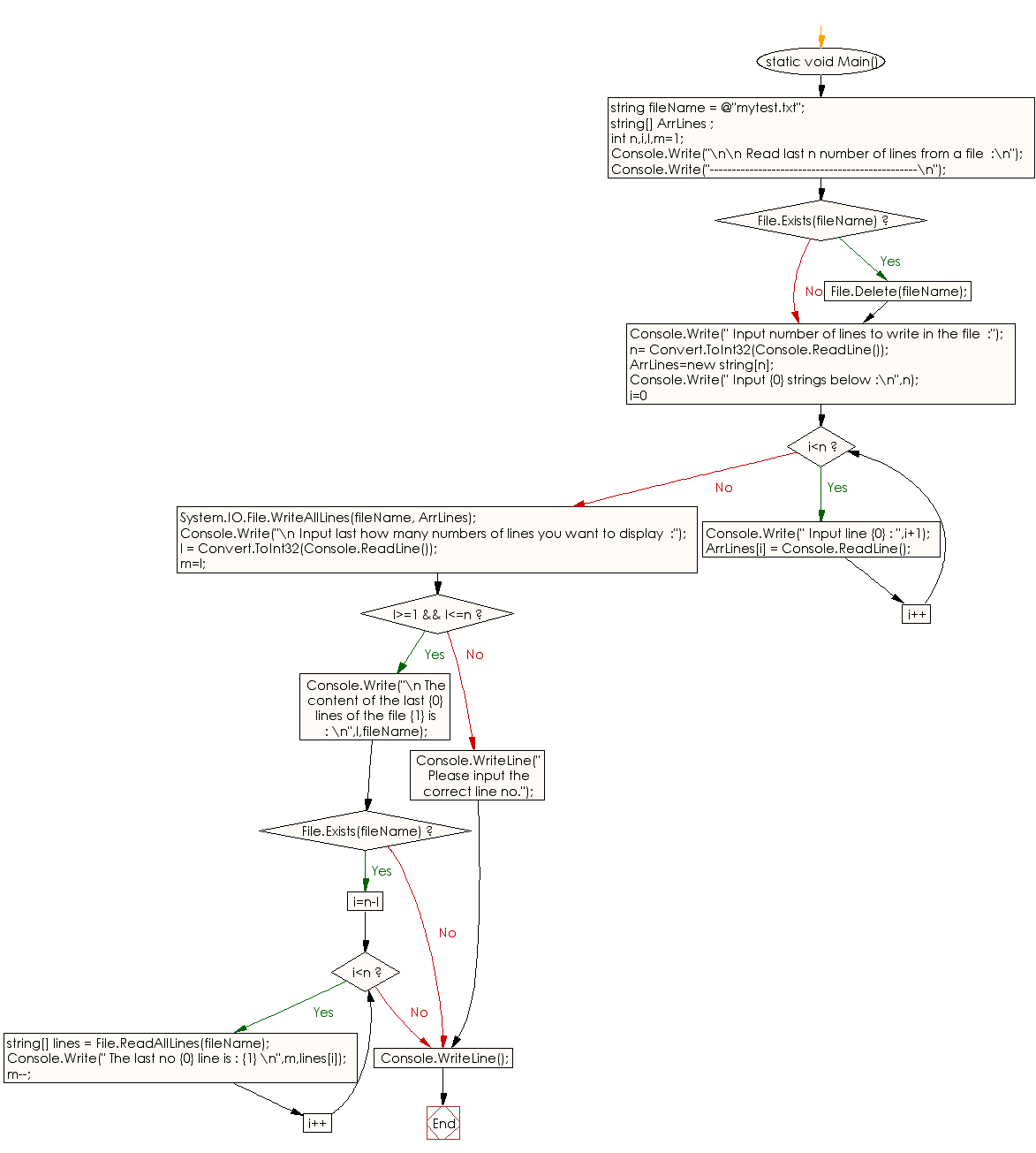
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to read a specific line from a file.
Next: Write a program in C# Sharp to count the number of lines in a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics