C#: Create and read the last line of a file
Write a program in C# Sharp to create and read the last line of a file.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace for basic functionalities
using System.IO; // Importing the System.IO namespace for file operations
class filexercise12 // Declaring a class named filexercise12
{
static void Main() // Declaring the Main method
{
string fileName = @"mytest.txt"; // Initializing a string variable with the file name
string[] ArrLines; // Declaring an array to store lines of text
int n, i; // Declaring variables for iteration and line count
// Displaying a message to create and read the last line of a file
Console.Write("\n\n Create and read the last line of a file :\n");
Console.Write("-----------------------------------------------\n");
if (File.Exists(fileName)) // Checking if the file exists and deleting it if true
{
File.Delete(fileName); // Deleting the file if it exists
}
Console.Write(" Input number of lines to write in the file :");
n = Convert.ToInt32(Console.ReadLine()); // Reading user input for the number of lines
ArrLines = new string[n]; // Initializing the array to store lines
Console.Write(" Input {0} strings below :\n", n); // Prompting user to input lines
for (i = 0; i < n; i++)
{
Console.Write(" Input line {0} : ", i + 1);
ArrLines[i] = Console.ReadLine(); // Reading lines of text from user input
}
// Writing all lines to the file
System.IO.File.WriteAllLines(fileName, ArrLines);
// Displaying the content of the last line of the file
Console.Write("\n The content of the last line of the file {0} is :\n", fileName);
if (File.Exists(fileName)) // Checking if the file exists
{
string[] lines = File.ReadAllLines(fileName); // Reading all lines from the file
Console.WriteLine(" {0}", lines[n - 1]); // Writing the last line of the file to the console
}
Console.WriteLine();
}
}
Sample Output:
Create and read the last line of a file : ----------------------------------------------- Input number of lines to write in the file :2 Input 2 strings below : Input line 1 : this is 1st line Input line 2 : this is 2nd line The content of the last line of the file mytest.txt is : this is 2nd line
Flowchart :
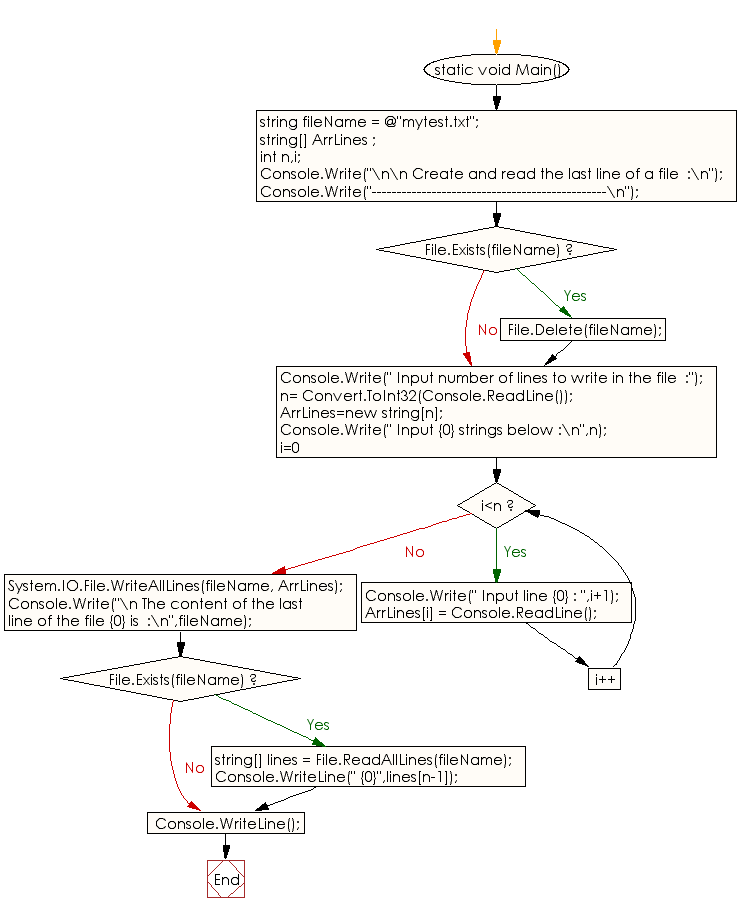
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to read the first line from a file.
Next:Write a program in C# Sharp to read a specific line from a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.