C#: Time of day from a given array of date time values
Write a C# Sharp program to get the time of day from a given array of date time values.
Sample Solution:-
C# Sharp Code:
using System;
public class Example7
{
// Main method, entry point of the program
public static void Main()
{
// Array of DateTime objects containing various dates and times
DateTime[] dates = {
DateTime.Now, // Current date and time
new DateTime(2016, 8, 16, 9, 28, 0), // August 16, 2016, 9:28 AM
new DateTime(2011, 5, 28, 10, 35, 0), // May 28, 2011, 10:35 AM
new DateTime(1979, 12, 25, 14, 30, 0) // December 25, 1979, 2:30 PM
};
// Iterate through each date in the dates array
foreach (var date in dates)
{
// Display the date portion of the DateTime object (without time)
// and the TimeOfDay portion (only the time)
Console.WriteLine("Day: {0:d} Time: {1:g}", date.Date, date.TimeOfDay);
// Display the date with its short time pattern (only time)
Console.WriteLine("Day: {0:d} Time: {0:t}\n", date);
}
}
}
Sample Output:
Day: 6/9/2017 Time: 18:34:55.787213 Day: 6/9/2017 Time: 6:34 PM Day: 8/16/2016 Time: 9:28:00 Day: 8/16/2016 Time: 9:28 AM Day: 5/28/2011 Time: 10:35:00 Day: 5/28/2011 Time: 10:35 AM Day: 12/25/1979 Time: 14:30:00 Day: 12/25/1979 Time: 2:30 PM
Flowchart :
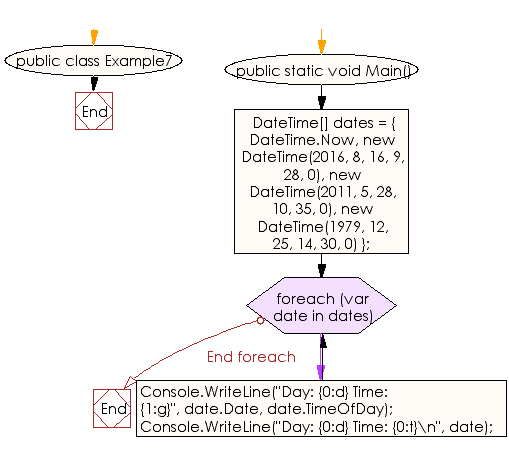
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to display the number of ticks that have elapsed since the beginning of the twenty-first century and to instantiate a TimeSpan object using the Ticks property.
Next: Write a C# Sharp program to retrieve the current date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.