C#: Display the number of ticks TimeSpan object using the Ticks property
Write a C# Sharp program to display the number of ticks that have elapsed since the beginning of the twenty-first century and to instantiate a TimeSpan object using the Ticks property.
Note: The TimeSpan object is then used to display the elapsed time using several other time intervals.
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
public class Example6
{
// Main method, entry point of the program
public static void Main()
{
// Get the current local date and time
DateTime localDate = DateTime.Now;
// Get the current UTC (Coordinated Universal Time) date and time
DateTime utcDate = DateTime.UtcNow;
// Array of culture names
String[] cultureNames = { "en-JM", "en-NZ", "fr-BE", "de-CH", "nl-NL" } ;
// Iterate through each culture name in the array
foreach (var cultureName in cultureNames)
{
// Create a CultureInfo object for the current culture name
var culture = new CultureInfo(cultureName);
// Display the native name of the culture
Console.WriteLine("{0}:", culture.NativeName);
// Display the local date and time in the current culture format
// and show the 'Kind' of the DateTime object (local or UTC)
Console.WriteLine(" Local date and time: {0}, {1:G}",
localDate.ToString(culture), localDate.Kind);
// Display the UTC date and time in the current culture format
// and show the 'Kind' of the DateTime object (always UTC)
Console.WriteLine(" UTC date and time: {0}, {1:G}\n",
utcDate.ToString(culture), utcDate.Kind);
}
}
}
Sample Output:
English (Jamaica): Local date and time: 09/06/2017 18:20:08, Local UTC date and time: 09/06/2017 12:50:08, Utc English (New Zealand): Local date and time: 9/06/2017 6:20:08 p.m., Local UTC date and time: 9/06/2017 12:50:08 p.m., Utc français (Belgique): Local date and time: 09-06-17 18:20:08, Local UTC date and time: 09-06-17 12:50:08, Utc Deutsch (Schweiz): Local date and time: 09.06.2017 18:20:08, Local UTC date and time: 09.06.2017 12:50:08, Utc Nederlands (Nederland): Local date and time: 9-6-2017 18:20:08, Local UTC date and time: 9-6-2017 12:50:08, Utc
Flowchart :
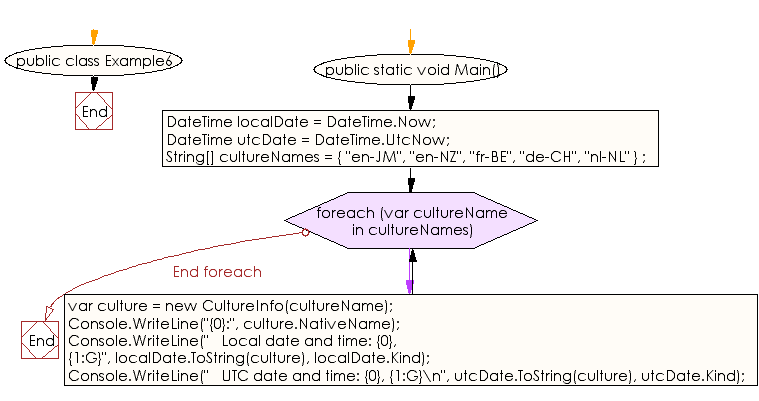
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to get a DateTime value that represents the current date and time on the local computer.
Next: Write a C# Sharp program to get the time of day from a given array of date time values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.