C#: Find the first day of the month against a given date
Write a program in C# Sharp to find the first day of the month against a given date.
Sample Solution:-
C# Sharp Code:
using System; // Importing the System namespace
class dttimeex54 // Declaring a class named dttimeex54
{
static void Main() // Declaring the Main method
{
int yr, mn, dt; // Declaring variables for year, month, and day
Console.Write("\n\n Find the first day of the month against a given date :\n"); // Displaying a message in the console
Console.Write("-----------------------------------------------------------\n"); // Displaying a separator line
// Input prompts for day, month, and year
Console.Write(" Input the Day : ");
dt = Convert.ToInt32(Console.ReadLine());
Console.Write(" Input the Month : ");
mn = Convert.ToInt32(Console.ReadLine());
Console.Write(" Input the Year : ");
yr = Convert.ToInt32(Console.ReadLine());
DateTime d = new DateTime(yr, mn, dt); // Creating a DateTime object with the provided year, month, and day
Console.WriteLine(" The formatted Date is : {0}", d.ToString("dd/MM/yyyy")); // Displaying the formatted input date
Console.WriteLine(" The first day of the month for the above date is : {0}\n", FirstDayOfMonth(d).ToString("dd/MM/yyyy")); // Displaying the first day of the month for the input date
}
// Method to find the first day of the month given a specific date
public static DateTime FirstDayOfMonth(DateTime dt)
{
return new DateTime(dt.Year, dt.Month, 1); // Creating a new DateTime object with the year and month from the input date and day set to 1 (first day of the month)
}
}
Sample Output:
Find the first day of the month against a given date : ----------------------------------------------------------- Input the Day : 12 Input the Month : 06 Input the Year : 2017 The formatted Date is : 12/06/2017 The first day of the month for the above date is : 01/06/2017
Flowchart:
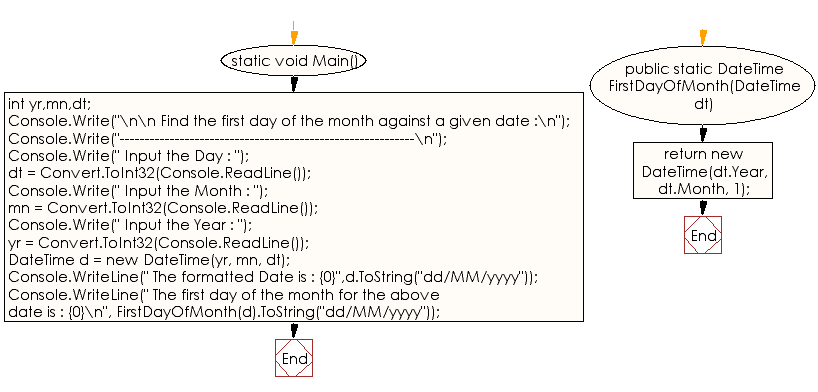
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to find the last day of a week against a given date.
Next: Write a program in C# Sharp to find the last day of a month against a given date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.