C#: Get the first day of the current year and first of a year against a given date
C# Sharp DateTime : Exercise-46 with Solution
Write a program in C# Sharp to get the first day of a year against a date.
C# Sharp Code:
using System;
class dttimeex46
{
static void Main()
{
int dt, mn, yr;
// Displaying the purpose of the program to the user.
Console.Write("\n\n Find the first day of a year against a date :\n");
Console.Write("---------------------------------------------------\n");
// Display the first day of the current year.
Console.WriteLine(" The First day of the current year is : {0} \n", FirstDayOfYear().ToString("dd/MM/yyyy"));
// Inputting a custom date from the user.
Console.Write(" Input the Day : ");
dt = Convert.ToInt32(Console.ReadLine());
Console.Write(" Input the Month : ");
mn = Convert.ToInt32(Console.ReadLine());
Console.Write(" Input the Year : ");
yr = Convert.ToInt32(Console.ReadLine());
// Creating a DateTime object with the provided day, month, and year.
DateTime d = new DateTime(yr, mn, dt);
Console.WriteLine(" The formatted Date is : {0}", d.ToString("dd/MM/yyyy"));
// Finding the first day of the year for the provided date.
DateTime nd = FirstDayOfYear(d);
Console.WriteLine(" The First day of the year {0} is : {1}\n", yr, nd.ToString("dd/MM/yyyy"));
}
// Method to find the first day of the year for the current date.
static DateTime FirstDayOfYear()
{
return FirstDayOfYear(DateTime.Today);
}
// Method to find the first day of the year for a given date.
static DateTime FirstDayOfYear(DateTime y)
{
return new DateTime(y.Year, 1, 1);
}
}
Sample Output:
Find the first day of a year against a date : --------------------------------------------------- The First day of the current year is : 01/01/2017 Input the Day : 12 Input the Month : 06 Input the Year : 2017 The formatted Date is : 12/06/2017 The First day of the year 2017 is : 01/01/2017
Flowchart :
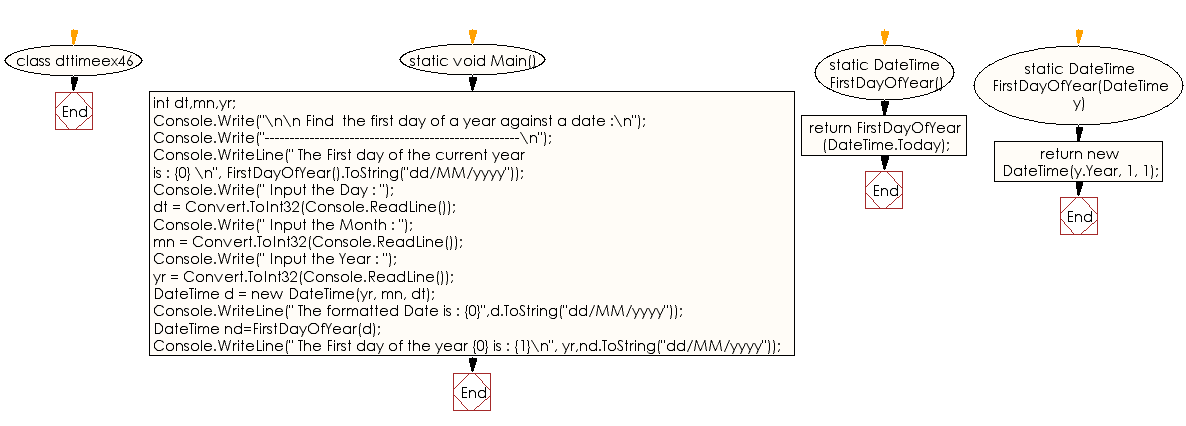
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a program in C# Sharp to compute what day will be tomorrow.
Next: Write a program in C# Sharp to get the last day of the current year against a given date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/datetime/csharp-datetime-exercise-46.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics