C#: Convert string representation of a date and time to its DateTime equivalent
Write a C# Sharp program to convert the specified string representation of a date and time to its DateTime equivalent.
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
public class Example41
{
public static void Main()
{
// Array of date and time strings in various formats.
string[] dateStrings = {"05/01/2016 14:57:32.8", "2016-05-01 14:57:32.8",
"2016-05-01T14:57:32.8375298-04:00", "5/01/2015",
"5/01/2015 14:57:32.80 -07:00",
"1 May 2015 2:57:32.8 PM", "16-05-2016 1:00:32 PM",
"Fri, 15 May 2016 20:10:57 GMT" };
DateTime dateValue; // Declare a variable to store parsed DateTime values.
// Display the attempt to parse strings using the current culture.
Console.WriteLine("Attempting to parse strings using {0} culture.",
CultureInfo.CurrentCulture.Name);
// Loop through each date string and attempt to parse it to a DateTime object.
foreach (string dateString in dateStrings)
{
// Try to parse the string to a DateTime and store the result in dateValue.
if (DateTime.TryParse(dateString, out dateValue))
// If successful, display the parsed date, time, and the kind of DateTime object.
Console.WriteLine(" Converted '{0}' to {1} ({2}).", dateString,
dateValue, dateValue.Kind);
else
// If parsing fails, display that the string couldn't be parsed.
Console.WriteLine(" Unable to parse '{0}'.", dateString);
}
}
}
Sample Output:
Attempting to parse strings using en-US culture. Converted '05/01/2016 14:57:32.8' to 5/1/2016 2:57:32 PM (Unspecified). Converted '2016-05-01 14:57:32.8' to 5/1/2016 2:57:32 PM (Unspecified). Converted '2016-05-01T14:57:32.8375298-04:00' to 5/2/2016 12:27:32 AM (Local). Converted '5/01/2015' to 5/1/2015 12:00:00 AM (Unspecified). Converted '5/01/2015 14:57:32.80 -07:00' to 5/2/2015 3:27:32 AM (Local). Converted '1 May 2015 2:57:32.8 PM' to 5/1/2015 2:57:32 PM (Unspecified). Unable to parse '16-05-2016 1:00:32 PM'. Unable to parse 'Fri, 15 May 2016 20:10:57 GMT'.
Flowchart:
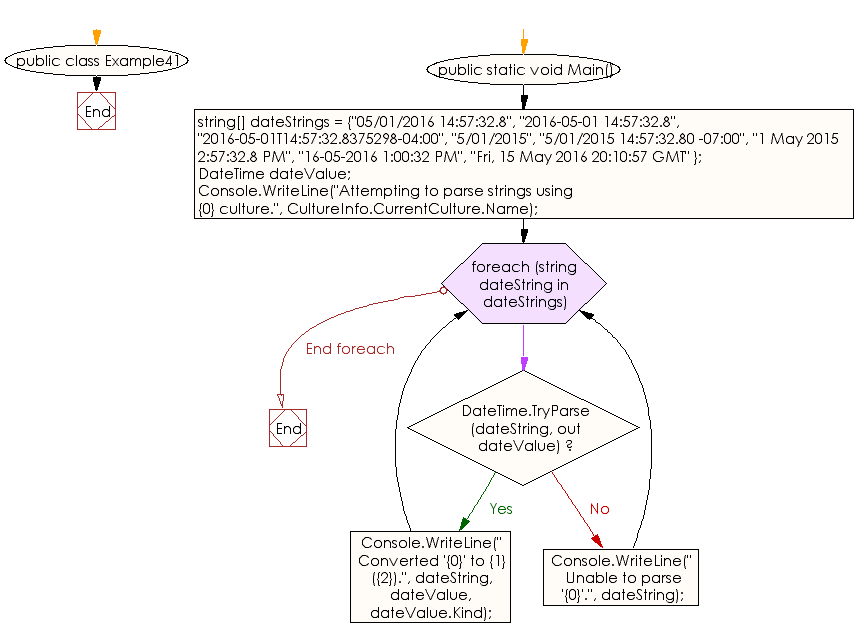
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert the value of the current DateTime object to Coordinated Universal Time (UTC).
Next: Write a C# Sharp program to convert the specified string representation of a date and time to its DateTime equivalent using the specified format, culture-specific format information, and style.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics