C#: Display the number of days of the year between two specified years
Write a C# Sharp program to display the number of days of the year between two specified years.
Sample Solution:-
C# Sharp Code:
using System;
public class Example4
{
// Main method, entry point of the program
public static void Main()
{
// Creating a DateTime object for December 31, 2000
DateTime dec31 = new DateTime(2000, 12, 31);
// Loop that adds years to the initial date and displays information for each iteration
for (int ctr = 0; ctr <= 20; ctr++)
{
// Adding 'ctr' years to the initial date (dec31)
DateTime dateToDisplay = dec31.AddYears(ctr);
// Displaying information about the current date:
// - The date itself in short date format {0:d}
// - The day of the year {1}
// - The year {2}
// - Checking if the year is a leap year and adding "(Leap Year)" if true
Console.WriteLine("{0:d}: day {1} of {2} {3}", dateToDisplay,
dateToDisplay.DayOfYear,
dateToDisplay.Year,
DateTime.IsLeapYear(dateToDisplay.Year) ?
"(Leap Year)" : "");
}
}
}
Sample Output:
12/31/2000: day 366 of 2000 (Leap Year) 12/31/2001: day 365 of 2001 12/31/2002: day 365 of 2002 12/31/2003: day 365 of 2003 12/31/2004: day 366 of 2004 (Leap Year) 12/31/2005: day 365 of 2005 12/31/2006: day 365 of 2006 12/31/2007: day 365 of 2007 12/31/2008: day 366 of 2008 (Leap Year) 12/31/2009: day 365 of 2009 12/31/2010: day 365 of 2010 12/31/2011: day 365 of 2011 12/31/2012: day 366 of 2012 (Leap Year) 12/31/2013: day 365 of 2013 12/31/2014: day 365 of 2014 12/31/2015: day 365 of 2015 12/31/2016: day 366 of 2016 (Leap Year) 12/31/2017: day 365 of 2017 12/31/2018: day 365 of 2018 12/31/2019: day 365 of 2019 12/31/2020: day 366 of 2020 (Leap Year)
Flowchart :
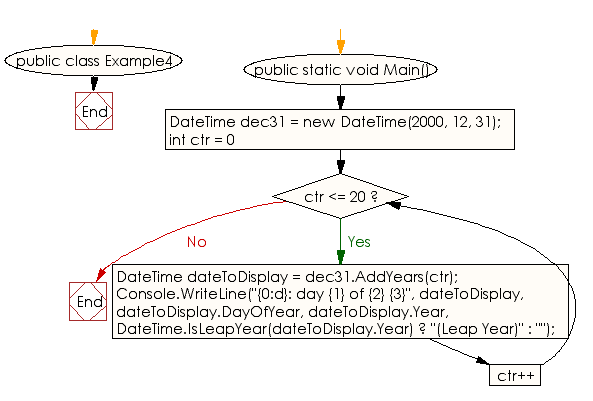
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to get the day of the week for a specified date.
Next: Write a C# Sharp program to get a DateTime value that represents the current date and time on the local computer.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.