C#: String representation of a date and time using CultureInfo objects
Write a C# Sharp program to display the string representation of a date and time using CultureInfo objects that represent five different cultures.
Sample Solution:-
C# Sharp Code:
Sample Output:
In Invariant Language (Invariant Country), 05/17/2016 09:00:00 In en-ZA, 2016-05-17 09:00:00 AM In ko-KR, 2016-05-17 오전 9:00:00 In de-DE, 17.05.2016 09:00:00 In es-ES, 17/05/2016 9:00:00 In en-US, 5/17/2016 9:00:00 AM
Flowchart :
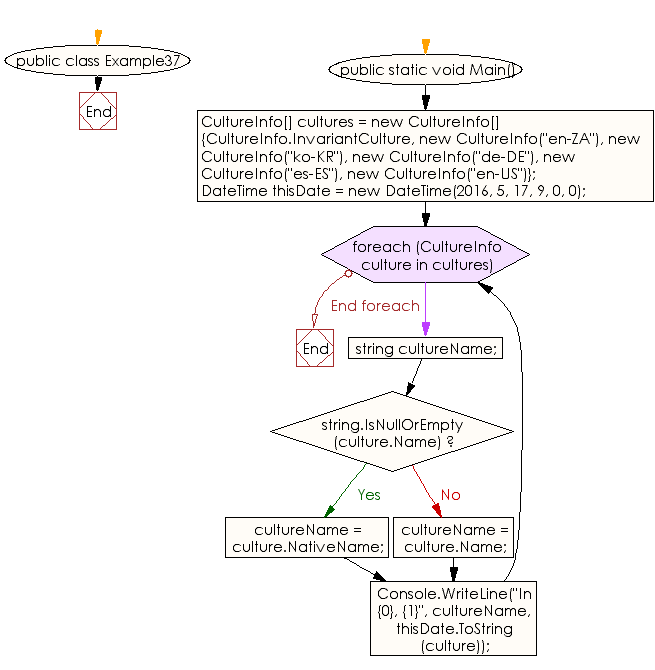
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert the value of the current DateTime object to its equivalent string representation using the formatting conventions of the current culture.
Next: Write a C# Sharp program to uses following three format strings to display a date and time value by using the conventions of the en-CA and sv-FI cultures.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics