C#: Convert current DateTime object to its equivalent string representation of current culture
Write a C# Sharp program to convert the current DateTime object value to its equivalent string representation. This is done using the current culture's formatting conventions.
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
using System.Threading;
public class Example36
{
public static void Main()
{
// Get the current culture
CultureInfo currentCulture = Thread.CurrentThread.CurrentCulture;
// Define a specific date and time
DateTime exampleDate = new DateTime(2016, 5, 16, 18, 32, 6);
// Display the date using the current (en-US) culture.
Console.WriteLine(exampleDate.ToString());
// Change the current culture to fr-FR and display the date.
Thread.CurrentThread.CurrentCulture = CultureInfo.CreateSpecificCulture("fr-FR");
Console.WriteLine(exampleDate.ToString());
// Change the current culture to ja-JP and display the date.
Thread.CurrentThread.CurrentCulture = CultureInfo.CreateSpecificCulture("ja-JP");
Console.WriteLine(exampleDate.ToString());
// Restore the original culture
Thread.CurrentThread.CurrentCulture = currentCulture;
}
}
Sample Output:
5/16/2016 6:32:06 PM 16/05/2016 18:32:06 2016/05/16 18:32:06
Flowchart:
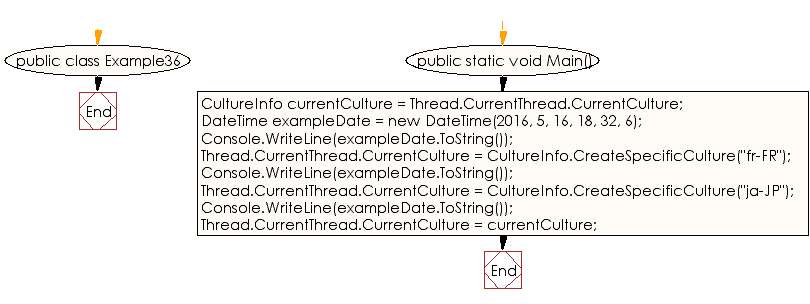
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert the value of the current DateTime object to its equivalent short time string representation.
Next: Write a C# Sharp program to display the string representation of a date and time using CultureInfo objects that represent five different cultures.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.