C#: Day of the week for a specified date
Write a C# Sharp program to get the day of the week for a specified date.
Sample Solution:-
C# Sharp Code:
using System;
public class Example3
{
// Main method, entry point of the program
public static void Main()
{
// Assume the current system is en-US.
// Creating a DateTime object representing July 11, 2016
DateTime dt = new DateTime(2016, 7, 11);
// Displaying the day of the week for the specified date
// Using {0:d} format to display the date in short date format
// Displaying the day of the week using the DayOfWeek property of the DateTime object
Console.WriteLine("The day of the week for {0:d} is {1}.", dt, dt.DayOfWeek);
}
}
Sample Output:
The day of the week for 7/11/2016 is Monday.
Flowchart :
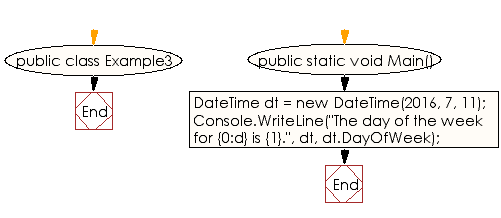
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to display the Day properties (year, month, day, hour, minute, second, millisecond etc.).
Next: Write a C# Sharp program to display the number of days of the year between two specified years.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics