C#: Converts date and time to its DateTime equivalent using the specified array of formats, culture-specific format information, and style
Write a program in C# Sharp to format a date and time of a specific string representation to its DateTime equivalent using the specified array of formats, culture-specific format information, and style.
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
public class Example29
{
public static void Main()
{
// Array of date and time formats
string[] formats= {
"M/d/yyyy h:mm:ss tt", "M/d/yyyy h:mm tt",
"MM/dd/yyyy hh:mm:ss", "M/d/yyyy h:mm:ss",
"M/d/yyyy hh:mm tt", "M/d/yyyy hh tt",
"M/d/yyyy h:mm", "M/d/yyyy h:mm",
"MM/dd/yyyy hh:mm", "M/dd/yyyy hh:mm",
"MM/d/yyyy HH:mm:ss.ffffff"
};
// Array of date strings to be converted
string[] dateStrings = {
"5/2/2009 6:32 PM", "05/02/2009 6:32:05 PM",
"5/2/2009 6:32:00", "05/02/2009 06:32",
"05/02/2009 06:32:00 PM", "05/02/2009 06:32:00",
"08/28/2016 16:17:39.125", "08/28/2016 16:17:39.125000"
};
DateTime dateValue; // Variable to hold parsed DateTime value
// Iterate through each date string in the array
foreach (string dateString in dateStrings)
{
try {
// Attempt to parse the date string using provided formats and culture (en-AU)
dateValue = DateTime.ParseExact(dateString, formats,
new CultureInfo("en-AU"),
DateTimeStyles.None);
// If successful, display the converted DateTime
Console.WriteLine("Converted '{0}' to {1}.", dateString, dateValue);
}
catch (FormatException) {
// If an exception occurs during parsing, display an error message
Console.WriteLine("Unable to convert '{0}' to a date.", dateString);
}
}
}
}
Sample Output:
Converted '5/2/2009 6:32 PM' to 5/2/2009 6:32:00 PM. Converted '05/02/2009 6:32:05 PM' to 5/2/2009 6:32:05 PM. Converted '5/2/2009 6:32:00' to 5/2/2009 6:32:00 AM. Converted '05/02/2009 06:32' to 5/2/2009 6:32:00 AM. Converted '05/02/2009 06:32:00 PM' to 5/2/2009 6:32:00 PM. Converted '05/02/2009 06:32:00' to 5/2/2009 6:32:00 AM. Unable to convert '08/28/2016 16:17:39.125' to a date. Converted '08/28/2016 16:17:39.125000' to 8/28/2016 4:17:39 PM.
Flowchart :
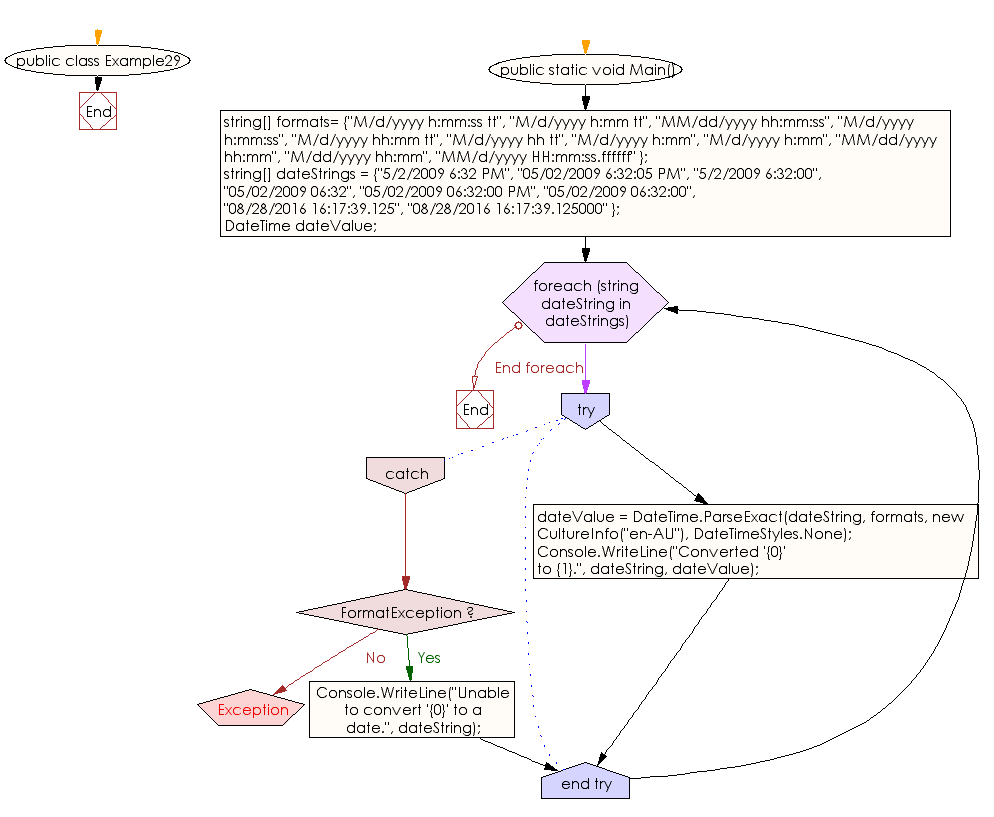
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to find the leap years between 1994 and 2016.
Next: Write a C# Sharp program to get the difference between two dates in days.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.