C#: Find the leap years between to specified years
C# Sharp DateTime : Exercise-28 with Solution
Write a C# Sharp program to find the leap years between 1994 and 2016.
Note : Use IsLeapYear method.
Sample Solution:-
C# Sharp Code:
using System;
public class Example28
{
public static void Main()
{
// Loop through years from 1995 to 2016
for (int year = 1995; year <= 2016; year++)
{
// Check if the current year is a leap year
if (DateTime.IsLeapYear(year))
{
// If it's a leap year, print that it is a leap year
Console.WriteLine("{0} is a leap year.", year);
// Create a DateTime object representing February 29 of the current year
DateTime leapDay = new DateTime(year, 2, 29);
// Get the date one year from the leap day and display it
DateTime nextYear = leapDay.AddYears(1);
Console.WriteLine(" One year from {0} is {1}.",
leapDay.ToString("d"),
nextYear.ToString("d"));
}
}
}
}
Sample Output:
1996 is a leap year. One year from 2/29/1996 is 2/28/1997. 2000 is a leap year. One year from 2/29/2000 is 2/28/2001. 2004 is a leap year. One year from 2/29/2004 is 2/28/2005. 2008 is a leap year. One year from 2/29/2008 is 2/28/2009. 2012 is a leap year. One year from 2/29/2012 is 2/28/2013. 2016 is a leap year. One year from 2/29/2016 is 2/28/2017.
Flowchart :
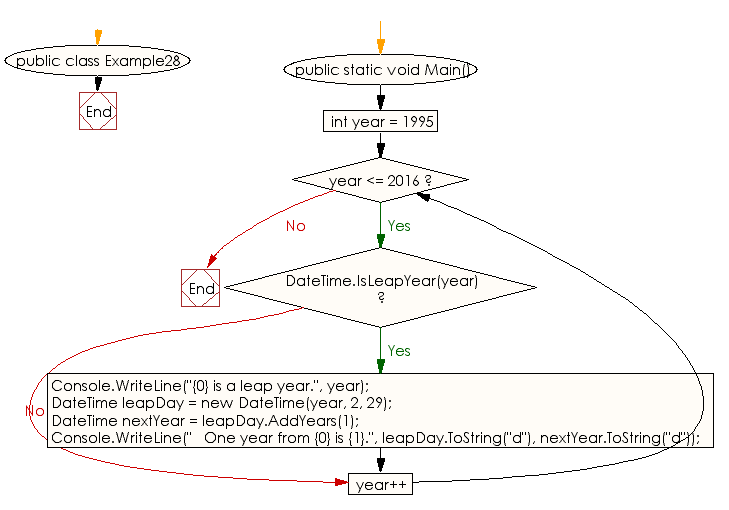
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to determine the type of a particular object.
Next: Write a C# Sharp program to convert the specified string representation of a date and time to its DateTime equivalent using the specified array of formats, culture-specific format information, and style.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/csharp-exercises/datetime/csharp-datetime-exercise-28.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics